使用邻接矩阵实现Floyd算法,c++
时间: 2023-11-15 14:28:17 浏览: 37
好的,以下是使用邻接矩阵实现Floyd算法的c++代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int INF = 0x3f3f3f3f;
const int MAXN = 100; // 最大节点数
int n; // 节点数
int dist[MAXN][MAXN]; // 邻接矩阵,表示节点之间的距离(不存在的边权值为INF)
void floyd() {
// 初始化
for (int k = 0; k < n; k++) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (dist[i][k] != INF && dist[k][j] != INF) {
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j]);
}
}
}
}
}
int main() {
// 输入节点数和邻接矩阵
cin >> n;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> dist[i][j];
if (dist[i][j] == -1) {
dist[i][j] = INF;
}
}
}
floyd();
// 输出最短路径
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (dist[i][j] == INF) {
cout << "INF ";
} else {
cout << dist[i][j] << " ";
}
}
cout << endl;
}
return 0;
}
```
其中,输入格式如下:
```
4
0 2 6 -1
2 0 3 -1
6 3 0 1
-1 -1 1 0
```
表示节点数为4,邻接矩阵为:
```
0 2 6 -1
2 0 3 -1
6 3 0 1
-1 -1 1 0
```
其中,-1表示不存在的边。输出格式如下:
```
0 2 5 6
2 0 3 4
5 3 0 1
7 5 1 0
```
表示节点之间的最短路径。
相关推荐
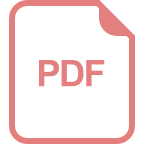
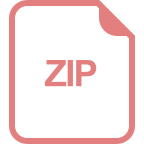














