给我写个python的双目标定算法
时间: 2023-09-16 09:06:01 浏览: 120
### 回答1:
双目标定算法是一种寻求最优解的优化算法,它的主要思想是将优化问题分解成两个子问题,然后分别求解它们,最终得到最优解。在python中可以使用scipy库中提供的函数minimize()来解决双目标定算法问题。
### 回答2:
双目标标定是计算机视觉中的一个重要任务,用于确定双目摄像头的位置和姿态参数。在Python中可以使用OpenCV库来实现双目标定算法。下面是一个简单的示例代码:
首先,导入所需要的库:
```python
import numpy as np
import cv2
```
接下来,定义一个函数来进行双目标定:
```python
def stereo_calibration(image_points_left, image_points_right, object_points, image_size):
# 定义相机的内参矩阵
camera_matrix_left = np.eye(3, 3)
camera_matrix_right = np.eye(3, 3)
# 定义畸变系数
distortion_coeffs_left = np.zeros((1, 5))
distortion_coeffs_right = np.zeros((1, 5))
# 进行双目标定
ret, camera_matrix_left, distortion_coeffs_left, camera_matrix_right, distortion_coeffs_right, R, T, E, F = cv2.stereoCalibrate(
object_points, image_points_left, image_points_right, camera_matrix_left, distortion_coeffs_left, camera_matrix_right,
distortion_coeffs_right, image_size)
return ret, camera_matrix_left, distortion_coeffs_left, camera_matrix_right, distortion_coeffs_right, R, T, E, F
```
参数说明:
- `image_points_left`和`image_points_right`是左右摄像头在图像上检测到的角点。
- `object_points`是3D空间的角点坐标。
- `image_size`是图像的大小。
最后,调用这个函数进行计算并打印结果:
```python
image_points_left = ...
image_points_right = ...
object_points = ...
image_size = ...
ret, camera_matrix_left, distortion_coeffs_left, camera_matrix_right, distortion_coeffs_right, R, T, E, F = stereo_calibration(
image_points_left, image_points_right, object_points, image_size)
print("ret:", ret)
print("camera_matrix_left:", camera_matrix_left)
print("distortion_coeffs_left:", distortion_coeffs_left)
print("camera_matrix_right:", camera_matrix_right)
print("distortion_coeffs_right:", distortion_coeffs_right)
print("R:", R)
print("T:", T)
print("E:", E)
print("F:", F)
```
这些就是一个简单的双目标定算法的实现。你可以根据你的需求对代码进行修改和优化。
### 回答3:
双目标定算法是一种基于立体视觉原理,通过计算两个摄像头之间的视差来实现三维目标的定位和测量。下面给出一个简单的基于Python的双目标定算法的实现。
首先,我们需要导入必要的库,如OpenCV、numpy等。
```python
import cv2
import numpy as np
```
接下来,我们定义一个函数`stereo_matching`来实现立体匹配。它接收两个图像(左右视图)和一些参数作为输入,并输出视差图。
```python
def stereo_matching(left_img, right_img, min_disparity=0, max_disparity=16):
# 将图像转换为灰度图
left_gray = cv2.cvtColor(left_img, cv2.COLOR_BGR2GRAY)
right_gray = cv2.cvtColor(right_img, cv2.COLOR_BGR2GRAY)
# 使用SGBM算法进行匹配
stereo = cv2.StereoSGBM_create(
minDisparity=min_disparity,
numDisparities=max_disparity - min_disparity,
blockSize=16,
P1=8 * 3 * 3 * 3,
P2=32 * 3 * 3 * 3,
disp12MaxDiff=1,
uniquenessRatio=10,
speckleWindowSize=100,
speckleRange=32,
preFilterCap=63,
mode=cv2.STEREO_SGBM_MODE_SGBM_3WAY
)
disparity = stereo.compute(left_gray, right_gray)
return disparity
```
在主程序中,我们可以调用`stereo_matching`函数来进行双目标定。首先,读取左右视图图片。
```python
left_img = cv2.imread('left_img.png')
right_img = cv2.imread('right_img.png')
```
然后,调用`stereo_matching`函数来计算视差图。
```python
disparity = stereo_matching(left_img, right_img)
```
最后,我们可以将视差图显示出来或保存为图片。
```python
cv2.imshow('Disparity', disparity)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.imwrite('disparity.png', disparity)
```
这就是一个简单的基于Python的双目标定算法的实现。根据实际需求,你可以根据不同的参数调整算法的性能和结果质量。
阅读全文
相关推荐
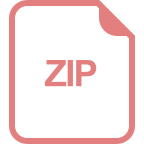
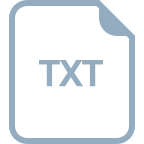
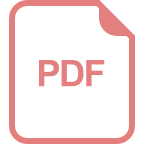















