def forward(self, x): x = self.fc1(x) x = self.act(x) x = self.drop(x) x = self.fc2(x) x = self.drop(x) return x
时间: 2023-09-09 11:12:50 浏览: 161
这段代码定义了模型的前向传播函数(`forward`)。在前向传播过程中,输入数据 `x` 经过一系列的层操作,最后返回输出结果。
具体的操作如下:
- `x = self.fc1(x)`:将输入 `x` 传递给第一个全连接层 `self.fc1`,进行线性变换。
- `x = self.act(x)`:将线性变换的结果 `x` 传递给激活函数层 `self.act`,进行非线性变换。
- `x = self.drop(x)`:将非线性变换的结果 `x` 传递给 Dropout 层 `self.drop`,在训练过程中随机丢弃一部分神经元。
- `x = self.fc2(x)`:将经过 Dropout 的结果 `x` 传递给第二个全连接层 `self.fc2`,进行线性变换。
- `x = self.drop(x)`:再次将线性变换的结果 `x` 传递给 Dropout 层 `self.drop`。
- 最后,返回经过全连接层和 Dropout 层处理后的结果 `x`。
这个前向传播函数定义了模型的计算流程,将输入数据经过一系列层的处理后得到最终的输出结果。在实际使用中,可以调用该函数来进行模型的前向计算。
相关问题
class Mlp(nn.Module): def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features self.fc1 = nn.Linear(in_features, hidden_features) self.act = act_layer() self.fc2 = nn.Linear(hidden_features, out_features) self.drop = nn.Dropout(drop) def forward(self, x): x = self.fc1(x) x = self.act(x) x = self.drop(x) x = self.fc2(x) x = self.drop(x) return x
这是一个 PyTorch 中定义的简单多层感知机(MLP)模型。下面是对这段代码的解释:
- `class Mlp(nn.Module):`:定义了一个名为 Mlp 的类,继承自 nn.Module,即是一个 PyTorch 模型。
- `def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):`:定义了 Mlp 类的构造函数,其中 `in_features` 表示输入特征的大小,`hidden_features` 表示隐藏层的大小(默认为 `in_features`),`out_features` 表示输出特征的大小(默认为 `in_features`),`act_layer` 表示激活函数(默认为 GELU),`drop` 表示 dropout 的概率(默认为 0)。
- `super().__init__()`:调用父类 nn.Module 的构造函数。
- `out_features = out_features or in_features`:如果没有指定 `out_features`,则将其设为 `in_features`。
- `hidden_features = hidden_features or in_features`:如果没有指定 `hidden_features`,则将其设为 `in_features`。
- `self.fc1 = nn.Linear(in_features, hidden_features)`:定义一个全连接层,输入大小为 `in_features`,输出大小为 `hidden_features`。
- `self.act = act_layer()`:定义一个激活函数,使用 `act_layer`。
- `self.fc2 = nn.Linear(hidden_features, out_features)`:定义一个全连接层,输入大小为 `hidden_features`,输出大小为 `out_features`。
- `self.drop = nn.Dropout(drop)`:定义一个 dropout 层,概率为 `drop`。
- `def forward(self, x):`:定义了模型的前向传播函数。
- `x = self.fc1(x)`:输入特征经过第一个全连接层。
- `x = self.act(x)`:激活函数。
- `x = self.drop(x)`:dropout。
- `x = self.fc2(x)`:输入特征经过第二个全连接层。
- `x = self.drop(x)`:dropout。
- `return x`:返回最终的输出特征。
class Mlp(nn.Module): """ MLP as used in Vision Transformer, MLP-Mixer and related networks """ def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features self.fc1 = nn.Linear(in_features, hidden_features) self.act = act_layer() self.drop1 = nn.Dropout(drop) self.fc2 = nn.Linear(hidden_features, out_features) self.drop2 = nn.Dropout(drop) def forward(self, x): x = self.fc1(x) x = self.act(x) x = self.drop1(x) x = self.fc2(x) x = self.drop2(x) return x
这是一个关于 MLP 的 PyTorch 模型的定义,用于 Vision Transformer、MLP-Mixer 等相关网络。它包括输入特征、隐藏特征、输出特征、激活函数、Dropout 等。在 forward 函数中,输入 x 经过两个全连接层和 Dropout 后输出。
阅读全文
相关推荐
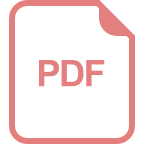
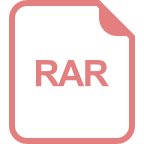



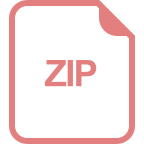
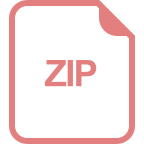
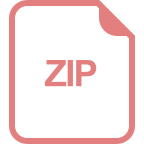
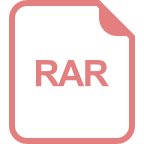
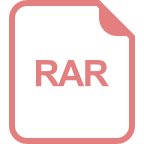