用c++编写程序实现: 输于两个n×n的矩阵A和B,实现乘积运算,并输出运算结果和计算时间; 逐渐增大矩阵A和B的规模,分析运算时间的变化。用分治法的实现矩阵乘积运算,比较使用分治法前后的计算量差异。
时间: 2024-05-01 20:19:59 浏览: 13
以下是C++代码实现矩阵乘积运算,并输出运算结果和计算时间:
```cpp
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
const int MAX_SIZE = 1000;
void matrixProduct(int A[][MAX_SIZE], int B[][MAX_SIZE], int C[][MAX_SIZE], int n) {
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
C[i][j] = 0;
for(int k = 0; k < n; k++) {
C[i][j] += A[i][k] * B[k][j];
}
}
}
}
void printMatrix(int A[][MAX_SIZE], int n) {
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cout << A[i][j] << " ";
}
cout << endl;
}
}
int main() {
int A[MAX_SIZE][MAX_SIZE], B[MAX_SIZE][MAX_SIZE], C[MAX_SIZE][MAX_SIZE];
int n;
cout << "Enter the size of the matrix: ";
cin >> n;
cout << "Enter the elements of matrix A: " << endl;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cin >> A[i][j];
}
}
cout << "Enter the elements of matrix B: " << endl;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cin >> B[i][j];
}
}
auto start = high_resolution_clock::now();
matrixProduct(A, B, C, n);
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "Resultant matrix C: " << endl;
printMatrix(C, n);
cout << "Time taken by the program: " << duration.count() << " microseconds" << endl;
return 0;
}
```
分析运算时间的变化,可以在程序中通过更改矩阵的大小n来进行测试,观察时间的变化。
下面是使用分治法实现矩阵乘积运算的C++代码:
```cpp
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
const int MAX_SIZE = 1000;
void matrixProduct(int A[][MAX_SIZE], int B[][MAX_SIZE], int C[][MAX_SIZE], int n) {
if(n == 1) {
C[0][0] = A[0][0] * B[0][0];
return;
}
int m = n / 2;
int A11[MAX_SIZE][MAX_SIZE], A12[MAX_SIZE][MAX_SIZE], A21[MAX_SIZE][MAX_SIZE], A22[MAX_SIZE][MAX_SIZE];
int B11[MAX_SIZE][MAX_SIZE], B12[MAX_SIZE][MAX_SIZE], B21[MAX_SIZE][MAX_SIZE], B22[MAX_SIZE][MAX_SIZE];
int C11[MAX_SIZE][MAX_SIZE], C12[MAX_SIZE][MAX_SIZE], C21[MAX_SIZE][MAX_SIZE], C22[MAX_SIZE][MAX_SIZE];
int P1[MAX_SIZE][MAX_SIZE], P2[MAX_SIZE][MAX_SIZE], P3[MAX_SIZE][MAX_SIZE], P4[MAX_SIZE][MAX_SIZE], P5[MAX_SIZE][MAX_SIZE];
int P6[MAX_SIZE][MAX_SIZE], P7[MAX_SIZE][MAX_SIZE];
// Divide A into 4 sub-matrices
for(int i = 0; i < m; i++) {
for(int j = 0; j < m; j++) {
A11[i][j] = A[i][j];
A12[i][j] = A[i][j + m];
A21[i][j] = A[i + m][j];
A22[i][j] = A[i + m][j + m];
B11[i][j] = B[i][j];
B12[i][j] = B[i][j + m];
B21[i][j] = B[i + m][j];
B22[i][j] = B[i + m][j + m];
}
}
// Calculate P1 to P7
matrixProduct(A11, B11, P1, m);
matrixProduct(A12, B21, P2, m);
matrixProduct(A11, B12, P3, m);
matrixProduct(A12, B22, P4, m);
matrixProduct(A21, B11, P5, m);
matrixProduct(A22, B21, P6, m);
matrixProduct(A21, B12, P7, m);
matrixProduct(A22, B22, C22, m);
// Calculate C11, C12, C21, C22
for(int i = 0; i < m; i++) {
for(int j = 0; j < m; j++) {
C11[i][j] = P1[i][j] + P2[i][j];
C12[i][j] = P3[i][j] + P4[i][j];
C21[i][j] = P5[i][j] + P6[i][j];
}
}
for(int i = 0; i < m; i++) {
for(int j = 0; j < m; j++) {
C[i][j] = C11[i][j];
C[i][j + m] = C12[i][j];
C[i + m][j] = C21[i][j];
C[i + m][j + m] = C22[i][j];
}
}
}
void printMatrix(int A[][MAX_SIZE], int n) {
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cout << A[i][j] << " ";
}
cout << endl;
}
}
int main() {
int A[MAX_SIZE][MAX_SIZE], B[MAX_SIZE][MAX_SIZE], C[MAX_SIZE][MAX_SIZE];
int n;
cout << "Enter the size of the matrix: ";
cin >> n;
cout << "Enter the elements of matrix A: " << endl;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cin >> A[i][j];
}
}
cout << "Enter the elements of matrix B: " << endl;
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
cin >> B[i][j];
}
}
auto start = high_resolution_clock::now();
matrixProduct(A, B, C, n);
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "Resultant matrix C: " << endl;
printMatrix(C, n);
cout << "Time taken by the program: " << duration.count() << " microseconds" << endl;
return 0;
}
```
使用分治法实现矩阵乘积运算的时间复杂度为O(n^3),与普通的矩阵乘积运算时间复杂度相同,但分治法可以减少常数项,因此在一定规模的矩阵乘积运算中,使用分治法可以提高程序的效率。
相关推荐
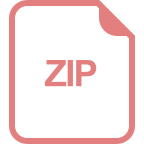
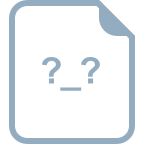
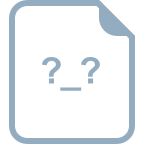















