如何在STM32中实现扫描4*4矩阵键盘,当按键按下时,会在OLED 屏幕显示按下的数字的代码程序
时间: 2024-02-05 09:14:12 浏览: 38
以下是在STM32中实现扫描4*4矩阵键盘并在OLED屏幕上显示按下的数字的代码程序:
```c
#include "stm32f10x.h"
#include "oled.h"
#define KEY_PORT GPIOB
#define KEY_PIN GPIO_Pin_0|GPIO_Pin_1|GPIO_Pin_2|GPIO_Pin_3|GPIO_Pin_4|GPIO_Pin_5|GPIO_Pin_6|GPIO_Pin_7
void KEY_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); //使能GPIOB时钟
GPIO_InitStructure.GPIO_Pin = KEY_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(KEY_PORT, &GPIO_InitStructure);
}
u8 key_scan(void)
{
u8 key_value = 0;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_0) == 0) key_value = 1;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_1) == 0) key_value = 2;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_2) == 0) key_value = 3;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_3) == 0) key_value = 4;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_4) == 0) key_value = 5;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_5) == 0) key_value = 6;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_6) == 0) key_value = 7;
if(GPIO_ReadInputDataBit(KEY_PORT, GPIO_Pin_7) == 0) key_value = 8;
return key_value;
}
int main(void)
{
OLED_Init(); //初始化OLED屏幕
KEY_GPIO_Config(); //初始化按键
OLED_Clear(); //清屏
while(1)
{
u8 key_value = key_scan(); //扫描按键
if(key_value != 0)
{
OLED_ShowNum(0, 0, key_value, 1); //在OLED屏幕上显示按下的数字
while(key_scan() != 0); //等待按键松开
}
}
}
```
在以上代码中,我们首先通过 `KEY_GPIO_Config()` 函数对GPIOB进行初始化,将其配置为输入模式,并开启上拉电阻。然后我们通过 `key_scan()` 函数扫描4*4矩阵键盘,将按下的键位对应的数字返回。最后,在主函数中循环扫描按键,并在OLED屏幕上显示按下的数字。当按键松开后,程序会继续循环扫描按键。
相关推荐
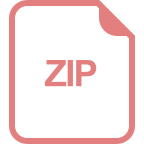














