cuckoo hash代码
时间: 2023-06-24 09:07:04 浏览: 61
以下是一个简单的C++实现Cuckoo Hash的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
const int TABLE_SIZE = 11;
const int MAX_RETRIES = 500;
class CuckooHash {
private:
vector<int> table1;
vector<int> table2;
public:
CuckooHash() {
table1.resize(TABLE_SIZE);
table2.resize(TABLE_SIZE);
}
int hash1(int key) {
return key % TABLE_SIZE;
}
int hash2(int key) {
return (key / TABLE_SIZE) % TABLE_SIZE;
}
void insert(int key) {
int count = 0;
while (count < MAX_RETRIES) {
int index1 = hash1(key);
int index2 = hash2(key);
if (table1[index1] == key || table2[index2] == key) {
cout << key << " already exists." << endl;
return;
}
if (table1[index1] == 0) {
table1[index1] = key;
return;
} else if (table2[index2] == 0) {
table2[index2] = key;
return;
} else {
int evictedKey = table1[index1];
table1[index1] = key;
key = table2[index2];
table2[index2] = evictedKey;
count++;
}
}
cout << "Insertion failed." << endl;
}
void remove(int key) {
int index1 = hash1(key);
int index2 = hash2(key);
if (table1[index1] == key) {
table1[index1] = 0;
} else if (table2[index2] == key) {
table2[index2] = 0;
} else {
cout << key << " does not exist." << endl;
}
}
bool search(int key) {
int index1 = hash1(key);
int index2 = hash2(key);
return table1[index1] == key || table2[index2] == key;
}
};
int main() {
CuckooHash hash;
hash.insert(10);
hash.insert(22);
hash.insert(37);
hash.insert(40);
hash.insert(50);
hash.insert(60);
hash.remove(22);
hash.remove(40);
hash.insert(70);
hash.insert(80);
cout << "Search for 10: " << (hash.search(10) ? "Found" : "Not Found") << endl;
cout << "Search for 37: " << (hash.search(37) ? "Found" : "Not Found") << endl;
cout << "Search for 50: " << (hash.search(50) ? "Found" : "Not Found") << endl;
cout << "Search for 80: " << (hash.search(80) ? "Found" : "Not Found") << endl;
cout << "Search for 90: " << (hash.search(90) ? "Found" : "Not Found") << endl;
return 0;
}
```
代码中使用了两个哈希表作为Cuckoo Hash的基础数据结构,其中使用了两个不同的哈希函数来计算每个键的两个哈希值。在插入键时,如果一个键已经存在于两个哈希表中的任何一个中,则插入操作失败。如果两个哈希表中有一个空闲位置,则将键插入其中一个哈希表中。如果两个哈希表中都没有空闲位置,则使用Cuckoo Hash算法将其中一个哈希表中的键替换为新插入的键,同时将被替换的键移动到另一个哈希表中。在删除键时,如果一个键存在于哈希表中,则将其从哈希表中删除。在搜索键时,如果一个键存在于两个哈希表中的任何一个中,则返回true,否则返回false。
相关推荐
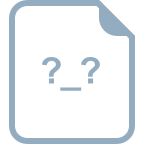














