numpy实现梯度下降
时间: 2023-08-31 21:14:29 浏览: 258
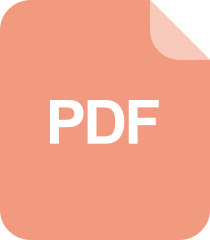
python+numpy+matplotalib实现梯度下降法
要使用NumPy实现梯度下降,首先需要定义一个损失函数和其对参数的梯度。然后,可以使用循环迭代的方式更新参数,直到达到收敛条件为止。
以下是一个使用NumPy实现梯度下降的示例代码:
```python
import numpy as np
# 定义损失函数
def loss_function(X, y, theta):
m = len(y)
h = X.dot(theta)
return np.sum((h - y) ** 2) / (2 * m)
# 定义梯度函数
def gradient(X, y, theta):
m = len(y)
h = X.dot(theta)
return X.T.dot(h - y) / m
# 定义梯度下降函数
def gradient_descent(X, y, theta, learning_rate, iterations):
m = len(y)
loss_history = []
for i in range(iterations):
grad = gradient(X, y, theta)
theta = theta - learning_rate * grad
loss = loss_function(X, y, theta)
loss_history.append(loss)
return theta, loss_history
# 生成随机数据
np.random.seed(0)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
# 添加偏置项
X_b = np.c_[np.ones((100, 1)), X]
# 初始化参数
theta_initial = np.random.randn(2, 1)
# 设置学习率和迭代次数
learning_rate = 0.01
iterations = 1000
# 运行梯度下降算法
theta_optimized, loss_history = gradient_descent(X_b, y, theta_initial, learning_rate, iterations)
print("Optimized theta:", theta_optimized)
```
在上面的示例代码中,我们首先定义了一个简单的线性回归问题,然后使用梯度下降算法来拟合参数。最终输出了优化后的参数theta。
请注意,上述示例代码仅用于演示梯度下降算法的实现过程,并不是最优化的实现方式。实际应用中,可以使用更高效的优化算法,如批量梯度下降、随机梯度下降或者使用优化库(如SciPy)中的函数来完成参数优化。
阅读全文
相关推荐
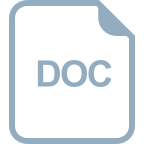
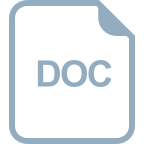


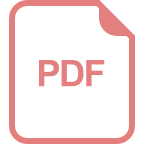

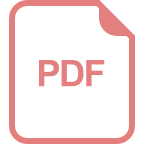
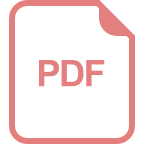







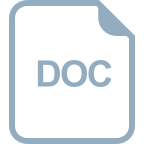
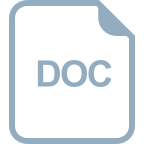
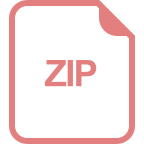
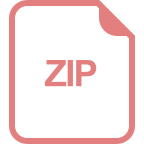