用c语言写一个基本坐标转换的函数库
时间: 2023-11-21 18:05:42 浏览: 154
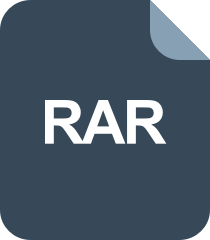
用C++编写的坐标转换源码

以下是一个基本的坐标转换函数库,包括平面直角坐标系、极坐标系和三维直角坐标系的转换:
```
#include <math.h>
// 平面直角坐标系转极坐标系
void cartesian_to_polar(double x, double y, double *r, double *theta)
{
*r = sqrt(x * x + y * y);
*theta = atan2(y, x);
}
// 极坐标系转平面直角坐标系
void polar_to_cartesian(double r, double theta, double *x, double *y)
{
*x = r * cos(theta);
*y = r * sin(theta);
}
// 三维直角坐标系转球面坐标系
void cartesian_to_spherical(double x, double y, double z, double *r, double *theta, double *phi)
{
*r = sqrt(x * x + y * y + z * z);
*theta = atan2(y, x);
*phi = atan2(sqrt(x * x + y * y), z);
}
// 球面坐标系转三维直角坐标系
void spherical_to_cartesian(double r, double theta, double phi, double *x, double *y, double *z)
{
*x = r * sin(phi) * cos(theta);
*y = r * sin(phi) * sin(theta);
*z = r * cos(phi);
}
```
使用示例:
```
#include <stdio.h>
int main()
{
double x = 3, y = 4, r, theta;
cartesian_to_polar(x, y, &r, &theta);
printf("cartesian (%f, %f) to polar: r = %f, theta = %f\n", x, y, r, theta);
polar_to_cartesian(r, theta, &x, &y);
printf("polar (r = %f, theta = %f) to cartesian: (%f, %f)\n", r, theta, x, y);
double x3 = 1, y3 = 2, z3 = 3, r3, theta3, phi3;
cartesian_to_spherical(x3, y3, z3, &r3, &theta3, &phi3);
printf("cartesian (%f, %f, %f) to spherical: r = %f, theta = %f, phi = %f\n", x3, y3, z3, r3, theta3, phi3);
spherical_to_cartesian(r3, theta3, phi3, &x3, &y3, &z3);
printf("spherical (r = %f, theta = %f, phi = %f) to cartesian: (%f, %f, %f)\n", r3, theta3, phi3, x3, y3, z3);
return 0;
}
```
阅读全文
相关推荐

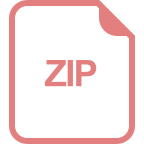
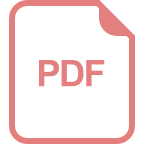
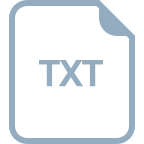
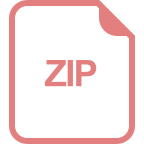
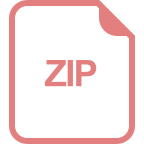
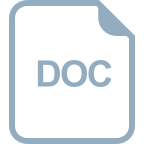
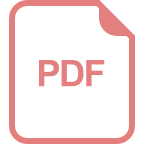
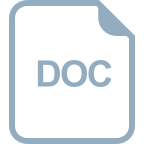
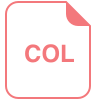
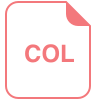




