c++用顺序表实现大整数的加法运算
时间: 2024-10-17 14:10:28 浏览: 67
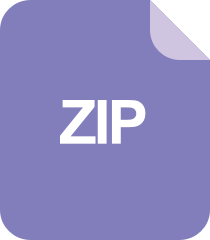
只需要用一张图片素材文档选择器.zip
在 C++ 中,使用顺序表(通常称为数组)实现大整数的加法运算,可以创建一个动态数组,每个元素代表一位数字,从高位到低位存储。由于直接操作数组会溢出整型范围,所以需要手动处理进位过程。
以下是基本步骤:
1. 定义一个 `BigInt` 类,包含一个动态数组 `digits` 存储每一位数字,以及两个指针 `index` 和 `carry` 分别表示当前操作的最高有效位和进位状态。
```cpp
class BigInt {
private:
int* digits;
size_t index;
int carry;
public:
// 构造函数、析构函数、获取/设置值等...
};
```
2. 实现 `BigInt` 的加法方法 `addBigInt`,接受另一个 `BigInt` 对象作为参数。循环遍历两个输入的序列,对于每一对数字加上进位,然后更新当前的索引和进位值:
```cpp
void BigInt::addBigInt(const BigInt& other) {
for (size_t i = 0; i < this->index || i < other.index; ++i) {
int sum = (*this->digits)[i] + (*other.digits)[i] + carry;
carry = sum / 10; // 计算并保存进位
(*this->digits)[i] = sum % 10; // 更新当前位置的数字
}
if (other.index > this->index) {
// 将其他数剩余部分追加到结果
while (other.index > this->index) {
this->digits[this->index++] = (*other.digits)[other.index - 1];
carry++;
}
} else if (this->index > other.index) {
// 同理,如果当前数更长,将剩余部分追加
while (this->index > other.index) {
this- 1];
carry++;
}
}
// 如果还有进位,将它加到最后一位
if (carry > 0) {
this->digits[this->index++] = carry;
}
}
```
3. 使用这个 `addBigInt` 方法时,先对两个 `BigInt` 进行初始化,然后调用 `addBigInt` 函数。
阅读全文
相关推荐
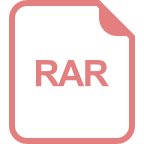
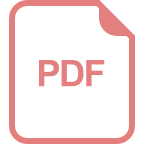
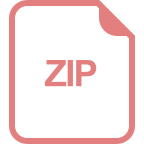
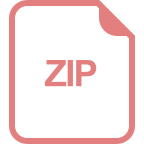
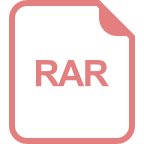
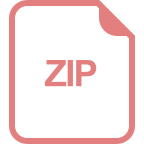
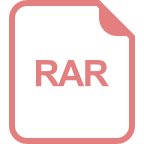
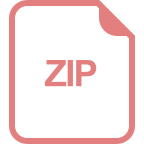
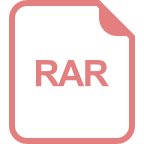
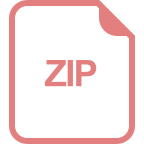