c++ unordered_map<int,int>dic;
时间: 2023-08-21 09:08:18 浏览: 200
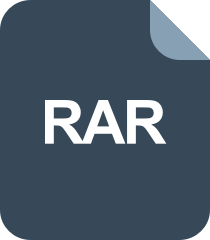
unordered-map的使用方法.rar
你好!关于你提到的C++的unordered_map<int, int> dic,我可以告诉你一些有关它的基本知识。
unordered_map是C++中的一个容器,用于存储键值对(key-value pairs)。在unordered_map中,每个键(key)都是唯一的,而值(value)可以重复。在你提供的代码中,unordered_map的键和值的类型都是int。
你可以通过以下方式使用unordered_map:
1. 插入键值对:
```cpp
dic.insert({key, value});
```
或者
```cpp
dic[key] = value;
```
2. 访问和修改值:
```cpp
int value = dic[key]; // 访问键为key的值
dic[key] = newValue; // 修改键为key的值为newValue
```
3. 检查键是否存在:
```cpp
if(dic.find(key) != dic.end()) {
// 键存在
}
```
4. 删除键值对:
```cpp
dic.erase(key); // 删除键为key的键值对
```
unordered_map提供了高效的查找和插入操作,其底层实现使用了哈希表。但需要注意的是,unordered_map不会按照插入的顺序来存储键值对。
希望这些信息能对你有所帮助!如果你还有其他问题,请继续提问。
阅读全文
相关推荐
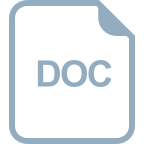
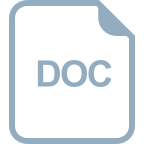















