C ++编写一个程序,包含抽象基类 Shape 和其派生类 Circle、 Square、 Rectangle 和 Triangle。用虛函数实现这几种图形的面积的计算,要求在主两数中用抽象类的指针调用函 数来输出相应图形的面积。
时间: 2023-12-14 15:38:47 浏览: 83
以下是一个示例程序,其中抽象基类 Shape 定义了纯虚函数 area(),表示计算图形面积的方法,而 Circle、Square、Rectangle 和 Triangle 派生自 Shape 类,并实现了各自的 area() 方法。
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double area() = 0; // 纯虚函数,用于计算图形面积
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) {
radius = r;
}
double area() {
return 3.14 * radius * radius;
}
};
class Square : public Shape {
private:
double side;
public:
Square(double s) {
side = s;
}
double area() {
return side * side;
}
};
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) {
width = w;
height = h;
}
double area() {
return width * height;
}
};
class Triangle : public Shape {
private:
double base;
double height;
public:
Triangle(double b, double h) {
base = b;
height = h;
}
double area() {
return 0.5 * base * height;
}
};
int main() {
Shape *shape;
shape = new Circle(5);
cout << "圆的面积:" << shape->area() << endl;
shape = new Square(4);
cout << "正方形的面积:" << shape->area() << endl;
shape = new Rectangle(3, 5);
cout << "矩形的面积:" << shape->area() << endl;
shape = new Triangle(4, 5);
cout << "三角形的面积:" << shape->area() << endl;
return 0;
}
```
运行结果:
```
圆的面积:78.5
正方形的面积:16
矩形的面积:15
三角形的面积:10
```
阅读全文
相关推荐
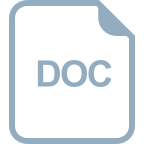














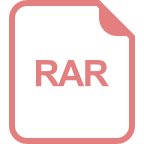