使用Python语言来接一元二次方程,并能实现画出函数坐标图
时间: 2024-09-26 10:13:33 浏览: 15
在Python中,可以使用内置的`numpy`和`matplotlib`库来处理一元二次方程并绘制函数图像。`numpy`用于数学计算,而`matplotlib`则用于图形展示。这里有一个简单的例子:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义一元二次方程函数
def quadratic_function(a, b, c):
def equation(x):
return a*x**2 + b*x + c
return equation
# 用户输入方程系数
a = float(input("请输入二次项系数(a): "))
b = float(input("请输入一次项系数(b): "))
c = float(input("请输入常数项(c): "))
# 解一元二次方程(这里仅用于演示,实际上可能需要引入numpy的linalg库)
def quadratic_solution(a, b, c):
delta = b ** 2 - 4 * a * c
if delta > 0:
x1 = (-b + np.sqrt(delta)) / (2 * a)
x2 = (-b - np.sqrt(delta)) / (2 * a)
return x1, x2
elif delta == 0:
x = -b / (2 * a)
return x, None
else: # 复数根
real_part = -b / (2 * a)
imaginary_part = np.sqrt(-delta) / (2 * a)
return complex(real_part, imaginary_part), complex(real_part, -imaginary_part)
solutions = quadratic_solution(a, b, c)
# 绘制函数图像
x_values = np.linspace(-10, 10, 400) # 创建x轴值的均匀分布
y_values = [quadratic_function(a, b, c)(x) for x in x_values]
plt.plot(x_values, y_values, label="y = " + str(a) + "x^2 + " + str(b) + "x + " + str(c))
if solutions[0] is not None:
plt.scatter([solutions[0]], [quadratic_function(a, b, c)(solutions[0])], color='red', marker='o')
plt.annotate('Root:', xy=(solutions[0], quadratic_function(a, b, c)(solutions[0])), xytext=(-20, 20),
textcoords='offset points', arrowprops=dict(facecolor='black', shrink=0.05))
plt.xlabel('x')
plt.ylabel('y')
plt.title('Quadratic Function Graph')
plt.legend()
plt.show()
相关推荐
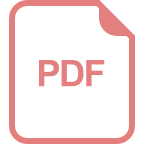
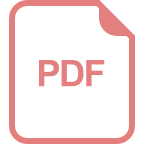
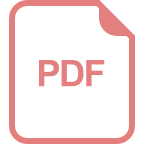

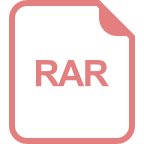
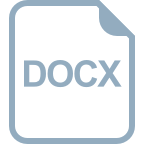
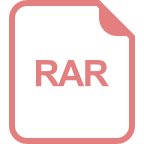
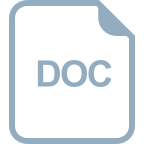
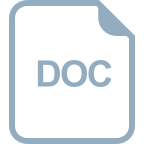
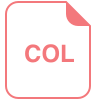
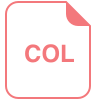

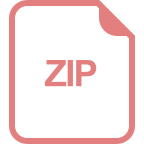
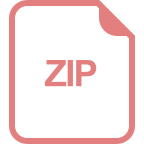
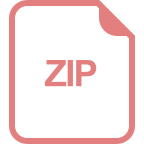
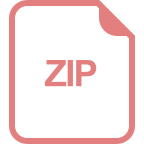
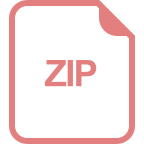