新建两个链表,一个无序,一个降序,然后将无序的链表插 入到降序的链表中,并使原来降序的链表仍然保持降序,输人的整 型数字以空格隔开。用c语言
时间: 2024-06-21 18:01:44 浏览: 11
在C语言中,你可以使用结构体来定义链表节点,然后创建两个链表,一个无序链表(`unordered_list`)和一个降序链表(`descending_list`)。插入操作可以通过遍历降序链表并在适当位置插入新节点来实现。这里是一个简单的步骤概述:
1. 定义链表节点结构体:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 初始化链表:
- 创建无序链表 `unordered_list` 和降序链表 `descending_list`。
- 读取输入,存储为整数数组。
```c
Node* unordered_list = NULL;
Node* descending_list = NULL;
int nums[] = { // 以空格隔开的输入数字
// ...
};
for (int i = 0; i < sizeof(nums) / sizeof(nums); i++) {
int num = nums[i];
Node* newNode = malloc(sizeof(Node));
newNode->data = num;
newNode->next = NULL;
if (!unordered_list) {
unordered_list = newNode;
} else {
// 插入到降序链表
Node* current = descending_list;
while (current && newNode->data > current->data) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
```
3. 插入无序链表中的节点到降序链表后,`descending_list` 会保持降序。
注意:以上代码仅是一个简化的概念示例,实际实现时需要处理边界情况(如链表为空)并确保内存管理(如`malloc`和`free`的使用)。
相关推荐
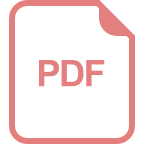
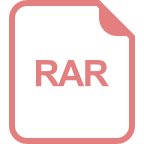














