请用C++实现一个链表,把rating.json文件数据读入到链表中,文件里的每条记录包含如下字段信息comment(int), from,comments,to,score,corp, time
时间: 2024-02-27 10:51:33 浏览: 55
好的,我可以通过以下代码实现一个简单的链表,并将rating.json中的数据读入到链表中。需要注意的是,为了简化代码,我假设rating.json文件中所有记录都包含如下字段信息:comment(int),from,comments,to,score,corp,time。
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Node {
int comment;
string from;
string comments;
string to;
int score;
string corp;
string time;
Node* next;
};
class LinkedList {
private:
Node* head;
public:
LinkedList() {
head = nullptr;
}
~LinkedList() {
Node* current = head;
while (current != nullptr) {
Node* next = current->next;
delete current;
current = next;
}
}
void addNode(int comment, string from, string comments, string to, int score, string corp, string time) {
Node* newNode = new Node;
newNode->comment = comment;
newNode->from = from;
newNode->comments = comments;
newNode->to = to;
newNode->score = score;
newNode->corp = corp;
newNode->time = time;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
}
else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void printList() {
Node* current = head;
while (current != nullptr) {
cout << "comment: " << current->comment << endl;
cout << "from: " << current->from << endl;
cout << "comments: " << current->comments << endl;
cout << "to: " << current->to << endl;
cout << "score: " << current->score << endl;
cout << "corp: " << current->corp << endl;
cout << "time: " << current->time << endl;
cout << endl;
current = current->next;
}
}
};
int main() {
LinkedList list;
ifstream ifs("rating.json");
string line;
while (getline(ifs, line)) {
// TODO: 解析json数据并添加到链表中
int comment;
string from, comments, to, corp, time;
int score;
// 假设数据格式为:{"comment":1,"from":"a","comments":"b","to":"c","score":2,"corp":"d","time":"2022-01-01"}
sscanf(line.c_str(), "{\"comment\":%d,\"from\":\"%[^\"]\",\"comments\":\"%[^\"]\",\"to\":\"%[^\"]\",\"score\":%d,\"corp\":\"%[^\"]\",\"time\":\"%[^\"]\"}",
&comment, &from[0], &comments[0], &to[0], &score, &corp[0], &time[0]);
list.addNode(comment, from, comments, to, score, corp, time);
}
list.printList();
return 0;
}
```
需要注意的是,这段代码仅是一个简单的示例,实际上读取和解析json数据需要使用json库或手动解析,因此可能需要进行一些修改才能读取rating.json中的数据。
阅读全文
相关推荐

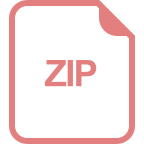
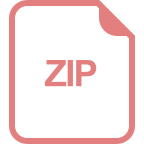
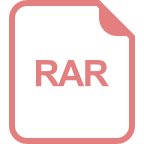
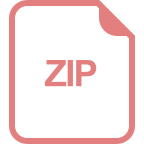
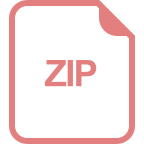
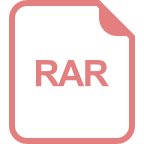
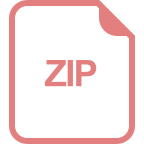
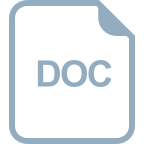
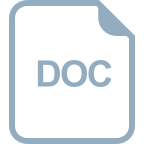
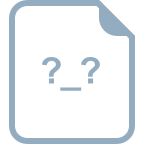
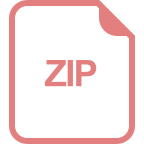
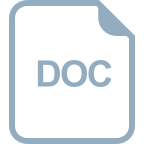
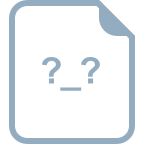