C++如何遍历一个文件夹中的文件
时间: 2023-05-29 10:03:41 浏览: 150
在C语言中,可以使用 dirent.h 头文件提供的函数来遍历一个文件夹中的文件。具体步骤如下:
1. 打开文件夹,使用 opendir() 函数,该函数会返回一个 DIR* 类型的指针,该指针指向打开的文件夹。
2. 使用 readdir() 函数,该函数会返回下一个文件或子文件夹的指针。每次调用该函数都会返回文件夹中的下一个文件或子文件夹,直到返回 NULL 为止。
3. 对于每个文件或子文件夹,使用 stat() 函数获取文件信息,例如文件名、文件大小等。
4. 处理完一个文件或子文件夹后,继续调用 readdir() 函数获取下一个文件或子文件夹,直到返回 NULL。
下面是一个遍历文件夹的示例代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <sys/stat.h>
int main(void) {
DIR *dir;
struct dirent *entry;
struct stat filestat;
dir = opendir(".");
if (dir == NULL) {
printf("Failed to open directory.\n");
return 1;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) { // 判断是否为普通文件
printf("File name: %s\n", entry->d_name);
stat(entry->d_name, &filestat);
printf("File size: %ld bytes\n", filestat.st_size);
}
}
closedir(dir);
return 0;
}
```
该代码会打开当前目录并遍历其中的所有普通文件,输出文件名和文件大小。需要注意的是,为了获取文件信息,需要使用 stat() 函数,并传入文件名作为参数。
相关推荐
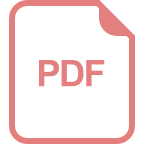
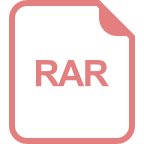














