C++读取txt文件,并将中文字符串保存到vector<char>中
时间: 2023-02-09 08:46:12 浏览: 249
可以使用C语言的文件操作函数fopen()、fread()、fclose()来读取txt文件,并使用STL中的vector<char>来保存读取的中文字符串。
示例代码如下:
```
#include <stdio.h>
#include <vector>
using namespace std;
int main() {
// 打开文件
FILE* fp = fopen("文件路径", "r");
if(fp == NULL) {
printf("文件打开失败!\n");
return -1;
}
// 定义存储字符串的vector
vector<char> v;
// 读取文件中的字符串
char c;
while((c = fgetc(fp)) != EOF) {
v.push_back(c);
}
// 关闭文件
fclose(fp);
// 遍历输出vector中存储的字符串
for(auto i : v) {
printf("%c", i);
}
return 0;
}
```
相关问题
C++读取txt文件,并仅将其中的中文字符串保存到vector<string>
### 回答1:
下面是一个示例代码:
```
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <locale>
using namespace std;
int main()
{
// 文件名
string fileName = "text.txt";
// 创建文件输入流
ifstream file(fileName);
// 设置locale,支持中文字符
file.imbue(locale("zh_CN.UTF-8"));
// 创建字符串变量
string line;
// 创建vector
vector<string> chineseStrings;
// 循环读取文件中的每一行
while (getline(file, line)) {
// 判断是否是中文字符串
if (line.find("[\u4e00-\u9fa5]+") != string::npos) {
// 如果是,添加到vector中
chineseStrings.push_back(line);
}
}
// 关闭文件
file.close();
// 输出vector中的所有字符串
for (string str : chineseStrings) {
cout << str << endl;
}
return 0;
}
```
请注意,上面的代码只是示例代码。在实际使用中可能需要进行更多的错误处理和调整。
### 回答2:
要读取一个txt文件并保存其中的中文字符串到一个vector<string>,你可以按照以下步骤进行操作:
1. 引入必要的头文件,包括iostream(输入输出流)、fstream(文件流)和vector(向量容器):
```cpp
#include <iostream>
#include <fstream>
#include <vector>
```
2. 创建一个函数来实现该功能:
```cpp
vector<string> getChineseStringsFromTxt(const string& filePath) {
vector<string> chineseStrings;
ifstream file(filePath);
if (file) {
string line;
while (getline(file, line)) {
string chineseString;
for (char c : line) {
if (isChineseChar(c)) { //判断字符是否为中文字符
chineseString += c;
}
}
if (!chineseString.empty()) {
chineseStrings.push_back(chineseString);
}
}
file.close();
}
else {
cout << "无法打开文件: " << filePath << endl;
}
return chineseStrings;
}
```
3. 创建一个辅助函数来判断字符是否为中文字符:
```cpp
bool isChineseChar(char c) {
return c >= -127 && c <= -2;
}
```
4. 在主函数中调用该函数并输出结果:
```cpp
int main() {
vector<string> result = getChineseStringsFromTxt("example.txt");
for (const string& chineseString : result) {
cout << chineseString << endl;
}
return 0;
}
```
以上是一个简单的实现方式,你可以根据自己的需求进行修改和调整。请记得首先将文本文件放在正确的位置,并传递正确的文件路径给函数。
### 回答3:
要读取txt文件并仅将其中的中文字符串保存到vector<string>,可以按照以下步骤进行:
1. 打开txt文件:使用C++中的fstream库,创建一个输入文件流对象,并使用open函数打开txt文件。例如:
```cpp
#include <fstream>
#include <string>
#include <vector>
std::ifstream file("example.txt");
if (!file.is_open()) {
// 文件打开失败,处理错误情况
}
```
2. 逐行读取文件内容:使用getline函数,循环读取文件的每一行。例如:
```cpp
std::string line;
while (std::getline(file, line)) {
// 处理每一行的内容
}
```
3. 提取中文字符串:对于每一行的内容,使用循环逐个字符判断是否为中文字符。可以使用C++中的ctype库中的iswalpha函数来判断一个字符是否为中文字符。若是中文字符,则将其添加到vector<string>中。例如:
```cpp
std::vector<std::string> chineseStrings;
for (char c : line) {
if (iswalpha(c)) {
std::string chCharacter(1, c); // 将字符转为字符串
chineseStrings.push_back(chCharacter);
}
}
```
4. 关闭文件:使用close函数关闭打开的文件流对象。例如:
```cpp
file.close();
```
以上就是将txt文件中的中文字符串保存到vector<string>的方法。最后,可以对vector<string>中保存的中文字符串进行进一步处理或使用。
C++中读取txt文件,并将其解析为vector<string>格式
### 回答1:
C++中可以使用fstream读取txt文件并解析到vector<string>中。
代码示例如下:
```c++
#include <fstream>
#include <string>
#include <vector>
using namespace std;
int main() {
vector<string> lines;
string line;
ifstream infile("example.txt");
while (getline(infile, line)) {
lines.push_back(line);
}
infile.close();
//遍历vector
for (int i = 0; i < lines.size(); i++) {
cout << lines[i] << endl;
}
return 0;
}
```
其中,"example.txt"是需要读取的文件名。
另外还可以使用 C standard library 中的 fopen 和 fgets 读取文件,解析到vector<string>中。
代码示例如下:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <vector>
using namespace std;
int main() {
vector<string> lines;
char line[1024];
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return(-1);
}
while (fgets(line, sizeof(line), file)) {
lines.push_back(line);
}
fclose(file);
//遍历vector
for (int i = 0; i < lines.size(); i++) {
printf("%s", lines[i].c_str());
}
return 0;
}
```
### 回答2:
在C语言中,要读取txt文件并将其解析为vector<string>格式,可以采用以下步骤:
1. 首先,需要包含相关的头文件,例如stdio.h和stdlib.h。这些头文件包含了一些用于文件操作的函数。
2. 创建一个FILE指针,用于打开和读取txt文件。可以使用fopen函数打开文件,并使用相应的模式(例如"r"表示只读方式打开文件)。
3. 创建一个vector<string>类型的变量,用于存储读取的文本行。可以使用vector容器来动态管理字符串数组。
4. 使用fgets函数从文件中逐行读取文本数据。可以使用循环来逐行读取整个文件内容,直到文件结尾。
5. 在每次读取到的文本行后,将其存储到vector<string>变量中。可以使用push_back函数将字符串添加到vector的末尾。
6. 当文件读取完毕后,关闭文件指针,使用fclose函数关闭已打开的文件。
7. 最后,可以通过遍历vector<string>来输出读取到的文本行,或者在需要的地方对文本行进行进一步处理。
下面是一个简单的示例代码,演示了如何在C语言中实现以上步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <vector>
int main() {
FILE *fp;
char line[256];
std::vector<std::string> lines;
// 打开txt文件
fp = fopen("example.txt", "r");
if (fp == NULL) {
printf("无法打开文件\n");
return 1;
}
// 逐行读取文本数据
while (fgets(line, sizeof(line), fp)) {
// 存储文本行到vector中
lines.push_back(line);
}
// 关闭文件
fclose(fp);
// 输出读取到的文本行
for (int i = 0; i < lines.size(); i++) {
printf("第%d行:%s", i+1, lines[i].c_str());
}
return 0;
}
```
这样,在C语言中我们就可以读取txt文件并将其解析为vector<string>格式了。
### 回答3:
在C语言中读取txt文件并将其解析为vector<string>格式,可以通过以下步骤实现:
1. 打开文件:首先需要使用C语言的文件操作函数打开要读取的txt文件。可以使用fopen函数来完成此操作。例如,可以使用以下代码打开名为"example.txt"的文件:
```c
FILE* file = fopen("example.txt", "r");
```
2. 读取文件内容:使用fgets函数逐行读取文件的内容。可以使用一个字符数组(例如char buffer[256])来存储每行的内容。fgets函数每次读取一行,直到文件结束。例如,可以使用以下代码读取文件内容:
```c
char buffer[256];
while (fgets(buffer, sizeof(buffer), file)) {
// 进行处理
}
```
3. 解析内容为vector<string>格式:将读取的每行内容解析为string类型,并依次存储到vector<string>中。可以使用C++标准库的string类和vector容器来完成此操作。例如,可以使用以下代码将每行内容存储到vector<string>中:
```c
#include <vector>
#include <string>
std::vector<std::string> lines;
char buffer[256];
while (fgets(buffer, sizeof(buffer), file)) {
std::string line(buffer);
lines.push_back(line);
}
```
4. 关闭文件:完成文件的读取后,需要使用fclose函数关闭文件。例如,可以使用以下代码关闭文件:
```c
fclose(file);
```
通过以上步骤,可以在C语言中读取txt文件并将其解析为vector<string>格式。在解析之后,可以对vector<string>进行进一步的操作和处理。
阅读全文
相关推荐
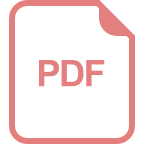
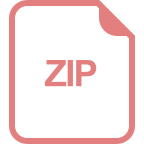
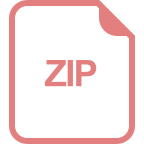













