使用上述MNIST数据集,选择合适的算法,编写程序,实现一个手写数字识别系统,并对实验结果进行分析,评价模型性能。
时间: 2024-03-17 20:39:41 浏览: 15
好的,针对您的需求,我可以给出一个基于深度学习的手写数字识别系统的实现过程,供您参考。
首先,我们需要使用Python中的TensorFlow或Keras等深度学习框架来建立一个卷积神经网络(CNN)模型。CNN是一种特殊的神经网络,它在图像处理和分析方面表现出色。我们可以使用TensorFlow中的tf.keras模块来构建CNN模型。
以下是一个简单的CNN模型结构:
```python
import tensorflow as tf
# 定义一个Sequential模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 打印模型结构
model.summary()
```
该模型包括两个卷积层和两个最大池化层。接下来是一个展平层(将输入数据从二维数组转换为一维数组)和两个全连接层,其中最后一个层使用了softmax激活函数以便输出10个分类结果。
接下来,我们需要加载MNIST数据集并对数据进行预处理。我们可以使用TensorFlow中的tf.keras.datasets模块来加载MNIST数据集,然后将图像数据标准化到0到1的范围内。
```python
# 加载MNIST数据集
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
# 将图像数据标准化到0到1的范围内
train_images = train_images.astype('float32') / 255.0
test_images = test_images.astype('float32') / 255.0
# 将标签数据转换为独热编码
train_labels = tf.keras.utils.to_categorical(train_labels)
test_labels = tf.keras.utils.to_categorical(test_labels)
# 将数据调整为CNN模型需要的输入格式
train_images = train_images.reshape((60000, 28, 28, 1))
test_images = test_images.reshape((10000, 28, 28, 1))
```
现在,我们可以编译并训练我们的CNN模型。在编译模型时,我们需要指定损失函数、优化器和评估指标。
```python
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
history = model.fit(train_images, train_labels, epochs=5, batch_size=64,
validation_data=(test_images, test_labels))
```
完成训练后,我们可以使用测试数据集来评估模型的性能。
```python
# 在测试数据集上评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
```
最后,我们可以使用训练好的模型来预测新的手写数字图像。
```python
# 加载一个手写数字图像
img = cv2.imread('test.png', 0)
img = cv2.resize(img, (28, 28))
img = np.array(img).reshape((1, 28, 28, 1)).astype('float32') / 255.0
# 使用训练好的模型进行预测
predictions = model.predict(img)
print(np.argmax(predictions[0]))
```
以上就是一个简单的手写数字识别系统的实现过程。您可以根据自己的需求和数据集进行修改和调整,以达到更好的性能和准确度。
相关推荐
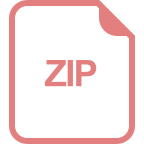
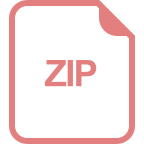
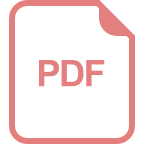














