#define _CRT_SECURE_NO_WARNINGS #include <stdlib.h> #include <stdio.h> #include <string.h> #include<ctype.h> void IsNotRepetition(char a[], char b[])//判断重复数量 { int i = 0, locate = 0, sum = 1; int len = strlen(a); while (i < len) { sum = 1; if (isdigit(a[i])) { sum = a[i] - '0'; i++; } for (int j = 0; j < sum; j++) { b[locate] = a[i]; locate++; } i++; } b[locate] = '\0'; } void IsRepetition(char a[], char b[])//判断重复 { int i = 0, count = 0, sum = 0; int len = strlen(a); while (i < len) { if (a[i] == a[i + 1]) count += 1; else { if (count == 1) { b[sum] = a[i]; sum++; } else { b[sum] = count; b[sum + 1] = a[i]; sum += 2; count = 1; } } i++; } b[sum] = '\0'; } void Compression(FILE* fp, FILE* fpl)//压缩指令 { char tran[1000], ch, res[1001]; int i = 0; fp = fopen("argv[1]","rb"); ch = fgetc(fp); while (ch != EOF) { tran[i] = ch; i++; } IsRepetition(tran, res); fclose(fp); //写入新文件 fpl = fopen("argv[3]", "wb"); fwrite(res, sizeof(char), strlen(res), fpl); fclose(fpl); } void Decompression(FILE* fp, FILE* fpl)//解压缩指令 { char tran[1000], ch, res[1001]; int i = 0; fp = fopen("argv[1]", "rb"); ch = fgetc(fp); while (ch != EOF) { tran[i] = ch; i++; } IsNotRepetition(tran, res); fclose(fp); //写入新文件 fpl = fopen("argv[3]", "wb"); fwrite(res, sizeof(char), strlen(res), fpl); fclose(fpl); } int main(int argc, char** argv) { if (strcmp(argv[2], "-c") == 0) { Compression(argv[1], argv[3]); } else if (strcmp(argv[2], "-d") == 0) { Decompression(argv[1], argv[3]); } return 0; }检查代码
时间: 2024-04-27 10:25:39 浏览: 13
这是一个文件压缩和解压缩程序的代码。程序接收命令行参数,第一个参数为要压缩或解压的文件路径,第二个参数为操作类型("-c"为压缩,"-d"为解压),第三个参数为处理结果输出的文件路径。
在压缩函数中,程序首先读取要处理的文件,然后调用函数IsRepetition对读取的文件进行压缩处理,将处理结果写入新文件。在解压函数中,程序也是首先读取要处理的文件,然后调用函数IsNotRepetition对读取的文件进行解压处理,将处理结果写入新文件。
该程序需要的头文件有stdlib.h、stdio.h、string.h和ctype.h。程序中实现了两个函数IsRepetition和IsNotRepetition,分别用于压缩和解压缩文件。在IsRepetition函数中,程序判断相邻字符是否相同,如果相同则计数器加1,如果不同则将计数器和当前字符写入结果数组(如果计数器为1,则直接写入字符)。在IsNotRepetition函数中,程序读取原始字符串,根据字符串中的数字来确定字符的重复数量,然后将字符写入结果数组中。
在main函数中,程序首先判断操作类型,然后调用相应的函数进行处理。
代码中有一条#define _CRT_SECURE_NO_WARNINGS,这是为了避免使用一些不安全的函数(如scanf)时产生编译警告而加入的。
相关问题
#define _CRT_SECURE_NO_WARNINGS #include <stdlib.h> #include <string.h> #include <stdio.h>
`#define _CRT_SECURE_NO_WARNINGS` 是一个预处理指令,用于禁用编译器对不安全函数的警告。在C/C++中,有一些函数被认为是不安全的,因为它们可能导致缓冲区溢出或其他安全问题。例如,`strcpy`、`scanf`等函数。当你使用这些函数时,编译器会发出警告,提醒你可能存在安全隐患。
通过在代码的开头添加 `#define _CRT_SECURE_NO_WARNINGS`,你可以告诉编译器不要发出这些警告。这在一些旧的代码或者特定的开发环境中可能会用到。
需要注意的是,禁用编译器警告可能会增加代码的风险,因此在使用这个预处理指令之前,最好先确保你的代码是安全的,并且了解禁用警告可能带来的潜在问题。
#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <string.h>
这段代码是C语言中常用的头文件和预处理指令,主要作用如下:
1. `_CRT_SECURE_NO_WARNINGS`:这是一个编译器预处理指令,用于禁止编译器产生某些警告信息。在C语言中,使用一些不安全的函数(如`strcpy`、`scanf`等)可能会导致安全问题,编译器会产生警告信息。使用该指令可以取消这些警告,但也可能会带来一定的安全隐患,因此需要慎重使用。
2. `stdio.h`:这是C语言中标准输入输出头文件,包含了一些常用的输入输出函数(如`printf`、`scanf`、`fopen`、`fclose`等)的声明和定义。
3. `stdlib.h`:这是C语言中标准库头文件,包含了一些常用的函数(如`malloc`、`free`、`atoi`、`exit`等)的声明和定义。
4. `string.h`:这是C语言中字符串操作头文件,包含了一些常用的字符串操作函数(如`strcpy`、`strcat`、`strlen`、`strcmp`等)的声明和定义。
综上,这段代码主要是为了使用标准输入输出函数、标准库函数和字符串操作函数,并取消编译器产生某些警告信息。
相关推荐
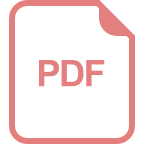
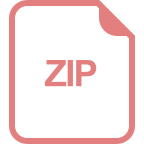
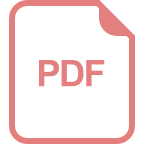













