整数四则运算表达式输出格式控制c++
时间: 2024-09-10 22:02:26 浏览: 80
在 C++ 中,控制整数四则运算表达式的输出格式通常涉及到 I/O流(Input/Output Stream)操作,特别是 `std::cout` 和 `std::endl`。当你需要按照特定格式打印计算结果时,可以使用格式化操纵符 `setw()` 来设置数字宽度,`setprecision()` 设置小数点后的位数,以及 `<<` 运算符插入值。
例如:
```cpp
#include <iostream>
#include <iomanip>
int main() {
int a = 123;
int b = 456;
// 输出整数部分居左,宽度为总长度
std::cout << std::setw(8) << "Result: " << a + b << std::endl;
// 或者只显示计算结果,保留两位小数
double result = static_cast<double>(a * b) / 100;
std::cout << "Division (with precision): " << std::fixed << std::setprecision(2) << result << std::endl;
return 0;
}
```
在这个例子中,`setw(8)` 指定“Result: ”字符串加上计算结果的总宽度为8字符。`std::fixed` 和 `std::setprecision(2)` 则用于固定小数点后有两位精度。
相关问题
栈实现非负整数四则运算计算器,写出c++代码
好的,以下是栈实现非负整数四则运算计算器的 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define STACK_SIZE 100 // 定义栈的大小
// 定义栈结构体
typedef struct {
int items[STACK_SIZE]; // 栈元素
int top; // 栈顶指针
} Stack;
// 初始化栈
void initStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack *s) {
return s->top == STACK_SIZE - 1;
}
// 入栈操作
void push(Stack *s, int num) {
if (isFull(s)) {
printf("栈已满,无法入栈。\n");
exit(1);
}
s->items[++(s->top)] = num;
}
// 出栈操作
int pop(Stack *s) {
if (isEmpty(s)) {
printf("栈为空,无法出栈。\n");
exit(1);
}
return s->items[(s->top)--];
}
// 获取栈顶元素
int peek(Stack *s) {
return s->items[s->top];
}
// 计算表达式的值
int calculate(char *expr) {
int i = 0, num = 0;
Stack numStack, opStack;
char c, op;
initStack(&numStack); // 初始化数字栈
initStack(&opStack); // 初始化操作符栈
while (expr[i] != '\0') {
c = expr[i];
if (isdigit(c)) { // 如果是数字
num = num * 10 + (c - '0');
if (!isdigit(expr[i+1])) { // 下一个字符不是数字,则表示数字已经结束
push(&numStack, num); // 将数字入栈
num = 0; // 将 num 置为零,用于下一个数字的计算
}
} else if (c == '+' || c == '-') { // 如果是加或减
if (isEmpty(&opStack)) { // 操作符栈为空,直接入栈
push(&opStack, c);
} else {
while (!isEmpty(&opStack) && peek(&opStack) != '(') { // 将所有高优先级的操作符出栈
op = pop(&opStack);
num = pop(&numStack);
if (op == '+') { // 执行加法操作
push(&numStack, pop(&numStack) + num);
} else { // 执行减法操作
push(&numStack, pop(&numStack) - num);
}
}
push(&opStack, c); // 将当前操作符入栈
}
} else if (c == '*' || c == '/') { // 如果是乘或除
if (isEmpty(&opStack) || peek(&opStack) == '+' || peek(&opStack) == '-') {
// 操作符栈为空或栈顶为低优先级操作符,直接入栈
push(&opStack, c);
} else {
// 将所有高优先级的操作符出栈
while (!isEmpty(&opStack) && (peek(&opStack) == '*' || peek(&opStack) == '/')) {
op = pop(&opStack);
num = pop(&numStack);
if (op == '*') { // 执行乘法操作
push(&numStack, pop(&numStack) * num);
} else { // 执行除法操作
push(&numStack, pop(&numStack) / num);
}
}
push(&opStack, c); // 将当前操作符入栈
}
} else if (c == '(') { // 如果是左括号,直接入栈
push(&opStack, c);
} else if (c == ')') { // 如果是右括号
while (!isEmpty(&opStack) && peek(&opStack) != '(') {
// 将所有括号内的操作符出栈
op = pop(&opStack);
num = pop(&numStack);
if (op == '+') { // 执行加法操作
push(&numStack, pop(&numStack) + num);
} else { // 执行减法操作
push(&numStack, pop(&numStack) - num);
}
}
pop(&opStack); // 将左括号出栈
}
i++;
}
// 将剩余的操作符出栈
while (!isEmpty(&opStack)) {
op = pop(&opStack);
num = pop(&numStack);
if (op == '+') { // 执行加法操作
push(&numStack, pop(&numStack) + num);
} else if (op == '-') { // 执行减法操作
push(&numStack, pop(&numStack) - num);
} else if (op == '*') { // 执行乘法操作
push(&numStack, pop(&numStack) * num);
} else if (op == '/') { // 执行除法操作
push(&numStack, pop(&numStack) / num);
}
}
return pop(&numStack); // 返回最终的结果
}
int main() {
char expr[100];
printf("请输入表达式:\n");
scanf("%s", expr);
printf("结果为:%d\n", calculate(expr));
return 0;
}
```
以上是栈实现非负整数四则运算计算器的 C 代码,使用方法为输入一个表达式并回车即可得到计算结果。
PTA求解简单的四则运算表达式
PTA(Programming Test and Assessment)平台上有很多简单的四则运算表达式题目,可以练习基本的算术运算和表达式求值。下面是一个例子:
题目描述:
给定一个只包含加、减、乘、除四种基本运算的表达式,求表达式的值。
输入格式:
第一行包含一个整数 T,表示共有 T 组测试数据。
每组数据占一行,包含一个长度不超过 50 的只包含数字与加、减、乘、除四种运算符的表达式。
输出格式:
对于每组数据,输出表达式的值,保留两位小数。
样例输入:
2
1+2*3-4/5
3+5*8-6/2
样例输出:
6.20
43.00
代码示例(C++):
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <iomanip>
using namespace std;
int main()
{
int T;
cin >> T;
while (T--)
{
string s;
cin >> s;
stack<double> nums;
stack<char> ops;
for (int i = 0; i < s.size(); i++)
{
if (isdigit(s[i]))
{
double num = s[i] - '0';
while (i + 1 < s.size() && isdigit(s[i + 1]))
{
num = num * 10 + s[i + 1] - '0';
i++;
}
nums.push(num);
}
else if (s[i] == '(')
{
ops.push('(');
}
else if (s[i] == ')')
{
while (ops.top() != '(')
{
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '+')
nums.push(a + b);
else if (op == '-')
nums.push(a - b);
else if (op == '*')
nums.push(a * b);
else if (op == '/')
nums.push(a / b);
}
ops.pop();
}
else if (s[i] == '+' || s[i] == '-')
{
while (!ops.empty() && (ops.top() == '+' || ops.top() == '-' || ops.top() == '*' || ops.top() == '/'))
{
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '+')
nums.push(a + b);
else if (op == '-')
nums.push(a - b);
else if (op == '*')
nums.push(a * b);
else if (op == '/')
nums.push(a / b);
}
ops.push(s[i]);
}
else if (s[i] == '*' || s[i] == '/')
{
while (!ops.empty() && (ops.top() == '*' || ops.top() == '/'))
{
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '+')
nums.push(a + b);
else if (op == '-')
nums.push(a - b);
else if (op == '*')
nums.push(a * b);
else if (op == '/')
nums.push(a / b);
}
ops.push(s[i]);
}
}
while (!ops.empty())
{
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if (op == '+')
nums.push(a + b);
else if (op == '-')
nums.push(a - b);
else if (op == '*')
nums.push(a * b);
else if (op == '/')
nums.push(a / b);
}
cout << fixed << setprecision(2) << nums.top() << endl;
}
return 0;
}
```
阅读全文
相关推荐
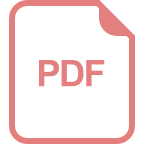
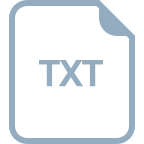
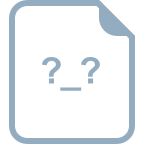
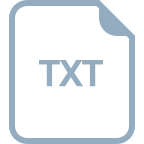
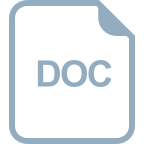



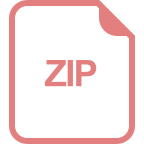
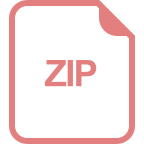
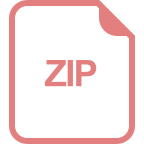
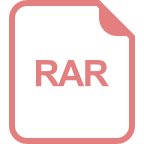
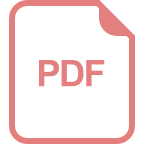