C代码关于创建无向图的邻接表存储结构
时间: 2023-08-20 17:07:13 浏览: 44
以下是一个基本的 C 代码示例,用于创建一个无向图的邻接表存储结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义邻接表节点
struct AdjListNode {
int dest;
struct AdjListNode* next;
};
// 定义邻接表
struct AdjList {
struct AdjListNode* head;
};
// 定义图
struct Graph {
int V;
struct AdjList* array;
};
// 创建邻接表节点
struct AdjListNode* newAdjListNode(int dest) {
struct AdjListNode* newNode = (struct AdjListNode*)malloc(sizeof(struct AdjListNode));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
// 创建图
struct Graph* createGraph(int V) {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
graph->V = V;
graph->array = (struct AdjList*)malloc(V * sizeof(struct AdjList));
int i;
for (i = 0; i < V; ++i) {
graph->array[i].head = NULL;
}
return graph;
}
// 添加边
void addEdge(struct Graph* graph, int src, int dest) {
struct AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
// 打印邻接表
void printGraph(struct Graph* graph) {
int v;
for (v = 0; v < graph->V; ++v) {
struct AdjListNode* pCrawl = graph->array[v].head;
printf("\n 邻接表节点 %d\n", v);
while (pCrawl) {
printf(" -> %d", pCrawl->dest);
pCrawl = pCrawl->next;
}
printf("\n");
}
}
// 测试主函数
int main() {
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
return 0;
}
```
这个示例代码创建了一个无向图,其中包含 5 个顶点,使用邻接表存储结构来表示。在主函数中,我们使用 `createGraph` 函数创建了一个空的图,并使用 `addEdge` 函数添加了一些边。最后,使用 `printGraph` 函数打印出了该图的邻接表表示形式。
输出结果应该如下:
```
邻接表节点 0
-> 4 -> 1
邻接表节点 1
-> 4 -> 3 -> 2 -> 0
邻接表节点 2
-> 3 -> 1
邻接表节点 3
-> 4 -> 2 -> 1
邻接表节点 4
-> 3 -> 1 -> 0
```
这个输出结果表示了每个顶点的邻居节点。例如,顶点 0 的邻居节点是 1 和 4。
相关推荐
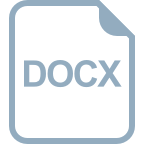
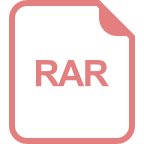
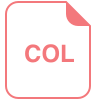













