python爬取yahoo图片
时间: 2023-11-09 14:48:59 浏览: 31
可以使用Python的第三方库requests和BeautifulSoup来实现爬取Yahoo图片的功能。具体步骤如下:
1. 使用requests发送请求,获取网页源代码。
2. 使用BeautifulSoup解析网页源代码,找到图片的地址。
3. 使用requests下载图片并保存到本地。
以下是示例代码:
```python
import requests
from bs4 import BeautifulSoup
# Yahoo图片的网址
url = 'https://www.yahoo.com/'
# 发送请求,获取网页源代码
response = requests.get(url)
html = response.text
# 解析网页源代码,找到图片的地址
soup = BeautifulSoup(html, 'html.parser')
img_tag = soup.find('img', class_='carousel__image')
img_url = img_tag['src']
# 下载图片并保存到本地
img_response = requests.get(img_url)
with open('yahoo.jpg', 'wb') as f:
f.write(img_response.content)
```
该代码将会下载Yahoo首页的轮播图并保存到本地,文件名为yahoo.jpg。注意,如果图片地址是相对路径,需要将其转换成绝对路径。
相关问题
python爬取雅虎图片
下面是一份Python代码,可以爬取Yahoo图片并保存到本地:
```python
import requests
from bs4 import BeautifulSoup
import urllib.request
url = "https://www.yahoo.com/news/photos"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
image_tags = soup.find_all("img")
for image_tag in image_tags:
image_url = image_tag.get("src")
if "https" in image_url:
image_name = image_url.split("/")[-1]
urllib.request.urlretrieve(image_url, image_name)
print(f"Image saved: {image_name}")
```
这段代码使用了Requests和BeautifulSoup库来获取网页内容和解析HTML。然后,它使用urllib库将图片保存到本地。
python爬取股票数据
好的,关于 python 爬取股票数据,您需要使用 Python 中的一些库来实现,比如 requests 和 BeautifulSoup 等库。以下是一个简单的 Python 程序,可用于爬取股票数据:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://finance.yahoo.com/quote/AAPL' #您要爬取的股票网站链接
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
price = soup.find('span', {'class': 'Trsdu(0.3s)'}).text
change = soup.find('span', {'class': 'Trsdu(0.3s)'}).find_next('span').text
print('Price: ' + price)
print('Change: ' + change)
```
这个程序首先使用 requests 库中的 get() 函数获取指定链接的 HTML 内容,然后使用 BeautifulSoup 库解析并提取 HTML 中的股票价格和变化,并将其打印出来。
当然,这只是一个简单的例子。实际上,爬取股票数据可能需要更复杂的程序,要考虑更多的细节。此外,涉及到数据的获取和使用,我们需要遵守一些法律法规和道德规范,以避免不必要的风险和损失。所以请注意使用。
相关推荐
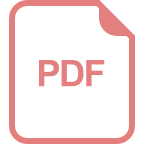
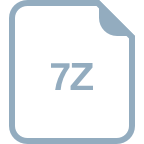
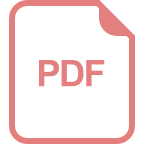
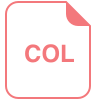











