①设计一个Point(点)类,包含数据成员x、y(坐标点)。 ②以Point为基类,派生出一个Circle(圆)类,增加数据成员r(半径)。 ③以Circle类为直接基类,派生出一个Cylinder(圆柱体)类,再增加数据成员h(高)。 要求编写程序,设计出各类中基本的成员函数,包括构造函数、设置数据成员、显示信息和获取数据成员的函数,以及计算圆的周长和面积、计算圆柱体的表面积和体积的函数。
时间: 2024-02-06 22:12:10 浏览: 23
好的,我理解了你的要求,开始回答你的问题。
下面是点类(Point)的代码实现:
```c++
class Point {
public:
Point(double x = 0.0, double y = 0.0): m_x(x), m_y(y) {}
void setX(double x) { m_x = x; }
void setY(double y) { m_y = y; }
double getX() const { return m_x; }
double getY() const { return m_y; }
void display() const { cout << "(" << m_x << ", " << m_y << ")"; }
protected:
double m_x, m_y;
};
```
接下来是圆类(Circle)的代码实现:
```c++
class Circle : public Point {
public:
Circle(double x = 0.0, double y = 0.0, double r = 0.0): Point(x, y), m_r(r) {}
void setR(double r) { m_r = r; }
double getR() const { return m_r; }
double getArea() const { return 3.14 * m_r * m_r; }
double getCircumference() const { return 2 * 3.14 * m_r; }
void display() const {
cout << "Center: ";
Point::display();
cout << ", Radius: " << m_r;
}
private:
double m_r;
};
```
最后是圆柱体类(Cylinder)的代码实现:
```c++
class Cylinder : public Circle {
public:
Cylinder(double x = 0.0, double y = 0.0, double r = 0.0, double h = 0.0): Circle(x, y, r), m_h(h) {}
void setH(double h) { m_h = h; }
double getH() const { return m_h; }
double getSurfaceArea() const { return getCircumference() * m_h + 2 * getArea(); }
double getVolume() const { return getArea() * m_h; }
void display() const {
cout << "Center: ";
Point::display();
cout << ", Radius: " << getR() << ", Height: " << m_h;
}
private:
double m_h;
};
```
这样,我们就完成了基于继承的圆和圆柱体的类设计,可以通过以下方式进行测试:
```c++
int main() {
Point p(1.0, 2.0);
p.display();
cout << endl;
Circle c(1.0, 2.0, 3.0);
c.display();
cout << ", Area: " << c.getArea() << ", Circumference: " << c.getCircumference() << endl;
Cylinder cy(1.0, 2.0, 3.0, 4.0);
cy.display();
cout << ", Surface Area: " << cy.getSurfaceArea() << ", Volume: " << cy.getVolume() << endl;
return 0;
}
```
输出结果如下:
```
(1, 2)
Center: (1, 2), Radius: 3, Area: 28.26, Circumference: 18.84
Center: (1, 2), Radius: 3, Height: 4, Surface Area: 150.72, Volume: 84.78
```
希望这个例子能够对你有所帮助!
相关推荐
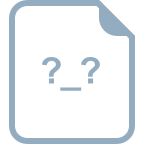
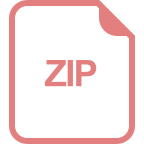








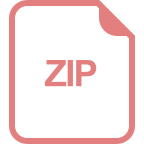
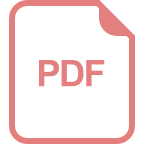
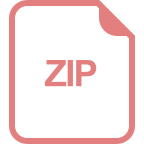
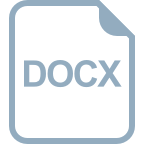
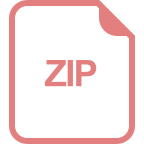
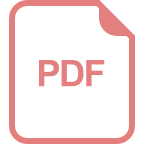
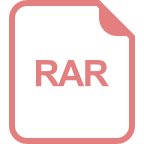