C++实现ffmpeg将图片推成rtsp视频流
时间: 2024-05-27 09:14:47 浏览: 427
要使用ffmpeg将图片推成RTSP视频流,需要按照以下步骤进行操作:
1. 安装ffmpeg
使用以下命令在Linux系统上安装ffmpeg:
sudo apt-get install ffmpeg
2. 准备图片
将需要转换为视频流的图片保存到本地。
3. 使用ffmpeg推流
使用以下命令将图片推成RTSP视频流:
ffmpeg -loop 1 -i [image_path] -f rtsp rtsp://[ip_address]:[port]/[stream_name]
其中,[image_path]是图片的本地路径,[ip_address]是本地IP地址,[port]是RTSP端口号,[stream_name]是视频流名称。
例如,将本地/home/user/image.jpg的图片推成RTSP视频流,IP地址为192.168.1.100,端口号为8554,流名称为test,使用以下命令:
ffmpeg -loop 1 -i /home/user/image.jpg -f rtsp rtsp://192.168.1.100:8554/test
4. 播放视频流
使用RTSP客户端软件(例如VLC)连接到RTSP服务器,输入URL rtsp://[ip_address]:[port]/[stream_name],即可播放视频流。
相关问题
ffmpeg将图片推成rtsp视频流c++
ffmpeg可以通过以下命令将图片推送为RTSP视频流:
```
ffmpeg -re -loop 1 -i image.jpg -f rtsp -rtsp_transport tcp rtsp://localhost:8554/stream
```
其中,-re选项表示以实时流的形式推送,-loop 1选项表示循环播放图片,-i选项指定输入的图片文件名,-f rtsp选项指定输出为RTSP格式,-rtsp_transport tcp选项指定RTSP使用TCP传输,rtsp://localhost:8554/stream是推送的RTSP流的地址。
请注意,这个命令只会循环播放一张图片,如果需要推送多张图片,可以使用ffmpeg的滤镜功能来实现。
c++ 使用ffmpeg4.x实现rtsp推流代码
下面是一个简单的使用FFmpeg 4.x实现RTSP推流的C++代码示例:
```c++
#include <iostream>
#include <cstdio>
#include <sstream>
#include <cstdlib>
#include <cstring>
#include <unistd.h>
#include <sys/time.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libswscale/swscale.h>
using namespace std;
#define WIDTH 640
#define HEIGHT 480
#define FPS 25
#define BITRATE 400000
#define RTSP_URL "rtsp://localhost:8554/test.sdp"
int main(int argc, char **argv) {
AVFormatContext *fmt_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVStream *stream = NULL;
AVFrame *frame = NULL;
AVPacket packet;
int ret = 0;
int frame_count = 0;
int64_t start_time = 0;
struct timeval tv;
int64_t pts = 0;
av_register_all();
avformat_network_init();
// 打开输出流
ret = avformat_alloc_output_context2(&fmt_ctx, NULL, "rtsp", RTSP_URL);
if (ret < 0) {
cout << "avformat_alloc_output_context2 failed: " << av_err2str(ret) << endl;
exit(1);
}
// 添加视频流
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
cout << "avcodec_find_encoder failed" << endl;
exit(1);
}
stream = avformat_new_stream(fmt_ctx, codec);
if (!stream) {
cout << "avformat_new_stream failed" << endl;
exit(1);
}
codec_ctx = stream->codec;
codec_ctx->codec_id = codec->id;
codec_ctx->bit_rate = BITRATE;
codec_ctx->width = WIDTH;
codec_ctx->height = HEIGHT;
codec_ctx->time_base = (AVRational){1, FPS};
codec_ctx->gop_size = 10;
codec_ctx->max_b_frames = 1;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
if (fmt_ctx->oformat->flags & AVFMT_GLOBALHEADER) {
codec_ctx->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
}
ret = avcodec_open2(codec_ctx, codec, NULL);
if (ret < 0) {
cout << "avcodec_open2 failed: " << av_err2str(ret) << endl;
exit(1);
}
av_dump_format(fmt_ctx, 0, RTSP_URL, 1);
ret = avio_open(&fmt_ctx->pb, RTSP_URL, AVIO_FLAG_WRITE);
if (ret < 0) {
cout << "avio_open failed: " << av_err2str(ret) << endl;
exit(1);
}
ret = avformat_write_header(fmt_ctx, NULL);
if (ret < 0) {
cout << "avformat_write_header failed: " << av_err2str(ret) << endl;
exit(1);
}
// 创建帧
frame = av_frame_alloc();
frame->width = WIDTH;
frame->height = HEIGHT;
frame->format = AV_PIX_FMT_YUV420P;
ret = av_frame_get_buffer(frame, 32);
if (ret < 0) {
cout << "av_frame_get_buffer failed: " << av_err2str(ret) << endl;
exit(1);
}
// 循环推流
while (true) {
gettimeofday(&tv, NULL);
if (start_time == 0) {
start_time = tv.tv_sec * 1000000 + tv.tv_usec;
}
pts = (tv.tv_sec * 1000000 + tv.tv_usec - start_time) * (double)FPS / 1000000;
frame->pts = pts;
// 生成测试图像
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
frame->data[0][y * frame->linesize[0] + x] = rand() % 256; // Y
}
}
for (int y = 0; y < HEIGHT / 2; y++) {
for (int x = 0; x < WIDTH / 2; x++) {
frame->data[1][y * frame->linesize[1] + x] = rand() % 256; // U
frame->data[2][y * frame->linesize[2] + x] = rand() % 256; // V
}
}
ret = avcodec_send_frame(codec_ctx, frame);
if (ret < 0) {
cout << "avcodec_send_frame failed: " << av_err2str(ret) << endl;
exit(1);
}
while (true) {
ret = avcodec_receive_packet(codec_ctx, &packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
cout << "avcodec_receive_packet failed: " << av_err2str(ret) << endl;
exit(1);
}
packet.stream_index = stream->index;
av_packet_rescale_ts(&packet, codec_ctx->time_base, stream->time_base);
ret = av_interleaved_write_frame(fmt_ctx, &packet);
if (ret < 0) {
cout << "av_interleaved_write_frame failed: " << av_err2str(ret) << endl;
exit(1);
}
av_packet_unref(&packet);
}
frame_count++;
if (frame_count >= FPS * 10) {
break; // 推送 10 秒
}
usleep(1000000 / FPS);
}
// 结束推流
av_write_trailer(fmt_ctx);
avcodec_close(codec_ctx);
avformat_free_context(fmt_ctx);
av_frame_free(&frame);
return 0;
}
```
这个程序会生成一些随机图像,并将它们推送到一个名为"test.sdp"的RTSP流中。你可以使用VLC等流媒体播放器来查看这个流。
阅读全文
相关推荐
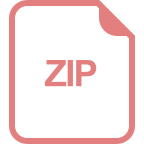
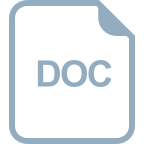
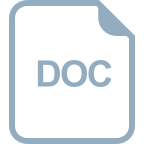








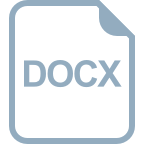