写一个用结构体当 key 的 std:: map 的 c++的例子
时间: 2023-09-04 15:04:15 浏览: 315
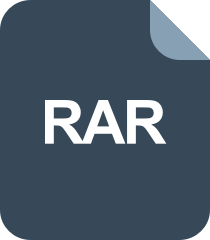
C++结构体简单例子
### 回答1:
可以的,以下是一个使用结构体作为 key 的 std::map 的 C++ 代码示例:
```c++
#include <iostream>
#include <map>
#include <string>
using namespace std;
struct Student {
string name;
int age;
double score;
bool operator<(const Student& other) const {
return name < other.name;
}
};
int main() {
map<Student, int> studentMap;
Student s1 = {"Tom", 18, 90.5};
Student s2 = {"Jerry", 19, 88.5};
Student s3 = {"Alice", 20, 95.};
studentMap[s1] = 1;
studentMap[s2] = 2;
studentMap[s3] = 3;
for (auto& it : studentMap) {
cout << it.first.name << " " << it.first.age << " " << it.first.score << " " << it.second << endl;
}
return ;
}
```
这个例子中,我们定义了一个结构体 `Student`,包含了学生的姓名、年龄和成绩。我们重载了 `<` 运算符,以便能够在 `map` 中使用结构体作为 key。然后我们创建了一个 `map`,将三个学生作为 key,对应的值分别为 1、2、3。最后我们遍历了这个 `map`,输出了每个学生的信息和对应的值。
### 回答2:
下面是一个使用结构体作为std::map的键的C++例子:
```cpp
#include <iostream>
#include <map>
// 声明一个结构体作为std::map的键
struct Key {
int x;
int y;
};
bool operator<(const Key& lhs, const Key& rhs) {
if (lhs.x != rhs.x) return lhs.x < rhs.x;
return lhs.y < rhs.y;
}
int main() {
std::map<Key, int> myMap;
// 向map中插入键值对
myMap.insert({{1, 2}, 10});
myMap.insert({{3, 4}, 20});
myMap.insert({{5, 6}, 30});
// 访问map中的元素
std::cout << "myMap[{1, 2}] = " << myMap[{1, 2}] << std::endl;
std::cout << "myMap[{5, 6}] = " << myMap[{5, 6}] << std::endl;
// 遍历map中的元素
for (const auto& pair : myMap) {
std::cout << "(" << pair.first.x << ", " << pair.first.y << "): " << pair.second << std::endl;
}
return 0;
}
```
这个例子中,我们定义了一个结构体`Key`作为`std::map`的键。为了让`std::map`正确比较这些结构体对象,我们重载了`<`操作符。在`main`函数中,我们创建了一个`myMap`对象,并向其中插入了一些键值对。我们可以通过索引访问map中的元素,并使用范围for循环遍历整个map并输出各个键和对应的值。
运行结果如下:
```
myMap[{1, 2}] = 10
myMap[{5, 6}] = 30
(1, 2): 10
(3, 4): 20
(5, 6): 30
```
### 回答3:
下面是一个使用结构体作为key的示例代码:
```c
#include <iostream>
#include <map>
#include <string>
struct Student {
std::string name;
int age;
double score;
// 重载小于运算符,用于比较结构体对象的排序
bool operator<(const Student& other) const {
if (name != other.name) {
return name < other.name;
} else if (age != other.age) {
return age < other.age;
} else {
return score < other.score;
}
}
};
int main() {
std::map<Student, std::string> studentMap;
// 创建几个学生对象作为key,对应学生姓名作为value
Student student1 = {"Alice", 18, 90.0};
Student student2 = {"Bob", 19, 80.0};
Student student3 = {"Alice", 18, 95.0};
// 插入键值对到map中
studentMap[student1] = "Class A";
studentMap[student2] = "Class B";
studentMap[student3] = "Class A";
// 打印map中的键值对
for (const auto& pair : studentMap) {
std::cout << "姓名: " << pair.first.name << ", 年龄: "
<< pair.first.age << ", 分数: "
<< pair.first.score << ", 班级: "
<< pair.second << std::endl;
}
return 0;
}
```
以上代码演示了如何使用结构体`Student`作为`std::map`的key,并且给每个学生对应一个班级。通过重载`<`运算符,可以实现结构体对象的比较和排序。最后,通过迭代器遍历`std::map`中的每个键值对,并输出对应的学生信息和班级。
阅读全文
相关推荐
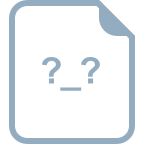
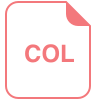
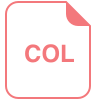
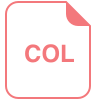



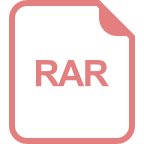
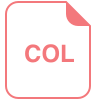
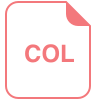
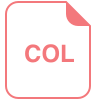
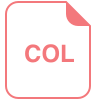
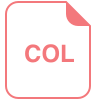



