java实现绘制空心半圆,空心圆形,空心矩形 ,空心三角形,空心梯形
时间: 2023-11-18 10:42:13 浏览: 188
可以使用Java的Graphics2D类来绘制空心图形。下面是一些例子:
1. 空心半圆:
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Arc2D;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class HalfCircle extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
Arc2D.Float arc = new Arc2D.Float(0, 0, width, height, 0, 180, Arc2D.OPEN);
g2d.draw(arc);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Half Circle");
frame.add(new HalfCircle());
frame.setSize(300, 300);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
2. 空心圆形:
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Ellipse2D;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Circle extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
Ellipse2D.Float ellipse = new Ellipse2D.Float(0, 0, width, height);
g2d.draw(ellipse);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Circle");
frame.add(new Circle());
frame.setSize(300, 300);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
3. 空心矩形:
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Rectangle2D;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Rectangle extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
Rectangle2D.Float rectangle = new Rectangle2D.Float(0, 0, width, height);
g2d.draw(rectangle);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Rectangle");
frame.add(new Rectangle());
frame.setSize(300, 300);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
4. 空心三角形:
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Polygon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Triangle extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
int[] xPoints = {0, width / 2, width};
int[] yPoints = {height, 0, height};
Polygon triangle = new Polygon(xPoints, yPoints, 3);
g2d.draw(triangle);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Triangle");
frame.add(new Triangle());
frame.setSize(300, 300);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
5. 空心梯形:
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Polygon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Trapezoid extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
int[] xPoints = {width / 4, 3 * width / 4, width, 0};
int[] yPoints = {0, 0, height, height};
Polygon trapezoid = new Polygon(xPoints, yPoints, 4);
g2d.draw(trapezoid);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Trapezoid");
frame.add(new Trapezoid());
frame.setSize(300, 300);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
阅读全文
相关推荐
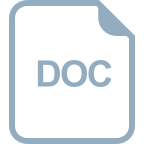
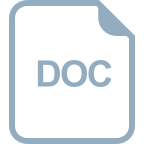
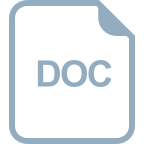
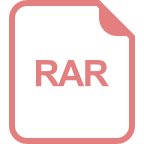
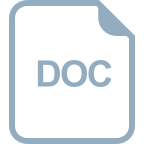
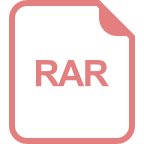
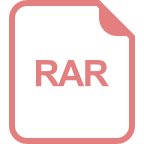
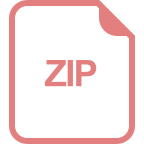
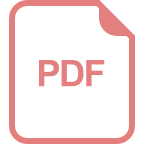
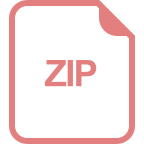
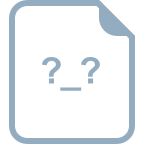
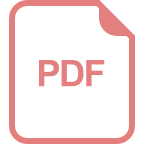
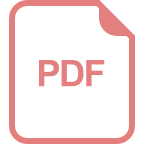
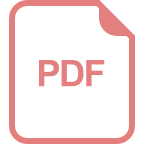
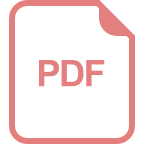
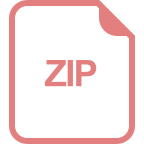
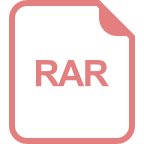