python与sqlalchemy连接
时间: 2023-10-16 22:09:10 浏览: 136
Python与SQLAlchemy的连接可以通过以下步骤进行:
1. 首先,确保已经安装了SQLAlchemy库。你可以使用以下命令来安装它:
```
pip install sqlalchemy
```
2. 导入所需的模块:
```python
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
```
3. 创建一个数据库引擎:
```python
engine = create_engine('数据库连接字符串')
```
这里的 '数据库连接字符串' 是指连接到你的数据库的字符串。具体的连接字符串取决于所使用的数据库类型和配置。
4. 创建一个会话工厂:
```python
Session = sessionmaker(bind=engine)
```
5. 使用会话工厂创建一个会话:
```python
session = Session()
```
通过这个会话对象,你可以执行SQLAlchemy提供的各种操作,如查询、插入、更新等。
请注意,以上步骤只是连接到数据库的基本操作。具体的数据库配置和连接字符串可能会有所不同,取决于你使用的数据库类型和设置。
相关问题
python使用sqlalchemy连接数据库
在Python中使用SQLAlchemy连接数据库需要经过以下几个步骤:
1. **安装与导入**[^1]:
```bash
pip install SQLAlchemy
```
安装完成后,通过`from sqlalchemy import create_engine, scoped_session, sessionmaker`来导入所需的模块。
2. **创建数据库引擎和连接池**:
```python
from sqlalchemy import create_engine
from sqlalchemy.pool import QueuePool
# 使用合适的数据库URL替换'db_url'
db_url = "your_database_connection_string"
engine = create_engine(db_url, poolclass=QueuePool)
# 创建一个带连接池的Session实例
Session = scoped_session(sessionmaker(bind=engine))
```
`db_url`应包含数据库类型、主机名、端口、数据库名称和其他必要信息。
3. **连接到数据库**:
```python
# 当需要时,调用Session.begin()开始一个新的事务
with Session() as session:
# 执行查询、插入、更新或删除操作
result = session.query(your_table).all()
session.add(your_new_object)
session.commit()
```
使用完毕后记得关闭连接,但通常在with语句中执行自动关闭。
python使用SQLAlchemy连接并操作国产化数据库高斯DB
Python使用SQLAlchemy连接和操作国产化数据库高斯DB(GaussDB),需要先安装相应的SQLAlchemy适配器,如`gaussdb-python`驱动。以下是基本步骤:
1. **安装依赖**:
安装SQLAlchemy库和特定的高斯DB驱动,例如:
```bash
pip install sqlalchemy psycopg2-binary # 如果是PostgreSQL风格的API
pip install pygaussdb # 针对GaussDB的官方适配器
```
2. **创建连接**:
使用SQLAlchemy的`create_engine`函数创建数据库引擎,提供数据库URL信息,例如:
```python
from sqlalchemy import create_engine
url = "postgresql://user:password@localhost:port/gaussdb_instance" # 替换为实际的数据库连接字符串
engine = create_engine(url)
```
或者如果使用的是gaussdb-python驱动:
```python
from pygaussdb.sqlalchemy import GaussDB dialect as gdialect
engine = create_engine(f'dialect+{gdialect}://user:password@localhost:port/dbname')
```
3. **操作数据库**:
- 创建Session对象用于数据库交互:
```python
from sqlalchemy.orm import sessionmaker
Session = sessionmaker(bind=engine)
session = Session()
```
- 执行查询、插入、更新和删除操作:
```python
# 查询数据
employees = session.query(User).filter_by(name='张三').all()
# 插入数据
new_employee = User(name='李四', email='li.si@example.com')
session.add(new_employee)
session.commit()
# 更新数据
employee = session.query(User).get(1)
employee.email = 'lisi@example.com'
session.commit()
# 删除数据
session.delete(employee)
session.commit()
```
4. **关闭连接**:
关闭Session以释放资源:
```python
session.close()
```
阅读全文
相关推荐





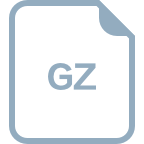










