用D3.js,写一个电路拓扑图
时间: 2024-03-02 17:52:51 浏览: 166
好的,以下是一个使用D3.js创建电路拓扑图的示例代码。在本例中,我们将创建一个简单的电路拓扑图,其中包含两个电阻和一个电池。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>D3.js电路拓扑图</title>
<script src="https://d3js.org/d3.v5.min.js"></script>
<style>
.wire {
stroke: black;
stroke-width: 2px;
}
.resistor {
fill: white;
stroke: black;
stroke-width: 2px;
}
.battery {
fill: white;
stroke: black;
stroke-width: 2px;
}
</style>
</head>
<body>
<svg width="400" height="200"></svg>
<script>
const svg = d3.select('svg');
const width = +svg.attr('width');
const height = +svg.attr('height');
// 定义电路元素的数据
const elements = [
{type: 'wire', x1: 50, y1: 100, x2: 150, y2: 100},
{type: 'wire', x1: 250, y1: 100, x2: 350, y2: 100},
{type: 'resistor', x: 150, y: 50},
{type: 'resistor', x: 150, y: 150},
{type: 'battery', x: 50, y: 50},
];
// 创建电路元素的组
const elementsGroup = svg.append('g')
.attr('class', 'elements');
// 添加电路元素到组中
elementsGroup.selectAll('.element')
.data(elements)
.enter()
.append('g')
.attr('class', 'element')
.attr('transform', d => `translate(${d.x},${d.y})`)
.each(function(d) {
const g = d3.select(this);
if (d.type === 'wire') {
g.append('line')
.attr('class', 'wire')
.attr('x1', d.x1)
.attr('y1', d.y1)
.attr('x2', d.x2)
.attr('y2', d.y2);
} else if (d.type === 'resistor') {
g.append('rect')
.attr('class', 'resistor')
.attr('x', -20)
.attr('y', -10)
.attr('width', 40)
.attr('height', 20);
} else if (d.type === 'battery') {
g.append('rect')
.attr('class', 'battery')
.attr('x', -20)
.attr('y', -10)
.attr('width', 40)
.attr('height', 20);
g.append('line')
.attr('class', 'wire')
.attr('x1', -20)
.attr('y1', 0)
.attr('x2', -30)
.attr('y2', 0);
g.append('line')
.attr('class', 'wire')
.attr('x1', 20)
.attr('y1', 0)
.attr('x2', 30)
.attr('y2', 0);
}
});
// 添加电路名称
svg.append('text')
.attr('x', width / 2)
.attr('y', 20)
.attr('text-anchor', 'middle')
.text('简单电路拓扑图');
</script>
</body>
</html>
```
在这个示例中,我们首先定义了电路元素的数据,它包含了两个电阻和一个电池。然后,我们创建了一个`<g>`元素用于存储所有电路元素,并使用`d3.select(this)`选择当前`<g>`元素来添加相应的SVG元素。最后,我们添加了电路名称,这个名称显示在SVG图形的中心。
希望这个示例能帮助您开始使用D3.js创建电路拓扑图。
阅读全文
相关推荐
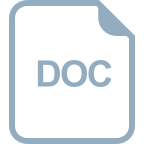
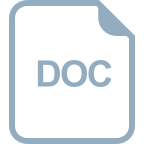
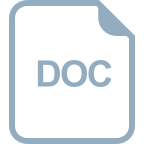

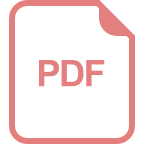
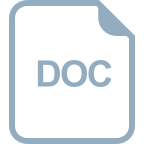
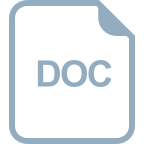


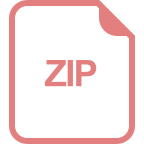
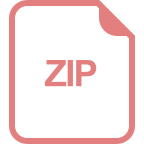
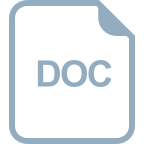
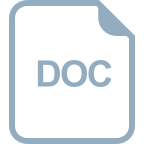
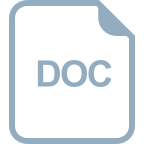
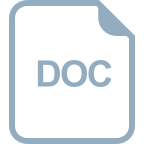
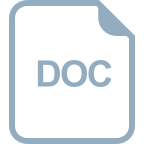
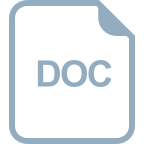

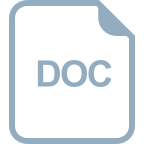