C++ priority_queue<vector<int>>heap要按造vector<int>容器内部的第一个元素组织为小根堆怎么操作
时间: 2024-06-02 11:03:42 浏览: 120
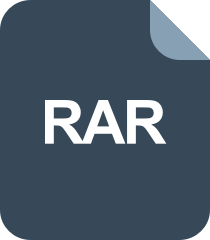
STL_quene.rar_crowd7oc_优先队列 堆_大根堆_大根对 c++_小根堆
要按照vector<int>容器内部的第一个元素组织为小根堆,可以通过自定义比较函数来实现。在C++的priority_queue中,默认情况下是按照元素的大小进行排序,但是我们可以通过自定义比较函数来改变排序规则。
首先,我们需要定义一个比较函数,用于比较两个vector<int>元素的大小。比较函数应该返回一个bool值,表示两个元素的大小关系。在这个比较函数中,我们只需要比较两个vector<int>的第一个元素即可。
接下来,我们可以使用priority_queue的构造函数来创建一个小根堆。在构造函数中,我们需要传入三个参数:容器类型、容器对象和比较函数。对于容器类型,我们可以使用vector<int>;对于容器对象,我们可以传入一个空的vector<int>;对于比较函数,我们传入自定义的比较函数即可。
下面是示例代码:
```cpp
#include <iostream>
#include <queue>
#include <vector>
// 自定义比较函数
struct Compare {
bool operator()(const std::vector<int>& a, const std::vector<int>& b) const {
return a[0] > b[0]; // 按照第一个元素升序排序
}
};
int main() {
std::priority_queue<std::vector<int>, std::vector<std::vector<int>>, Compare> heap;
// 向堆中插入元素
heap.push({3, 1, 4});
heap.push({1, 5, 9});
heap.push({2, 6, 5});
// 输出堆中的元素
while (!heap.empty()) {
std::vector<int> top = heap.top();
heap.pop();
for (int num : top) {
std::cout << num << " ";
}
std::cout << std::endl;
}
return ;
}
```
运行以上代码,输出结果为:
```
1 5 9
2 6 5
3 1 4
```
阅读全文
相关推荐
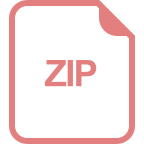






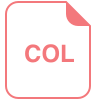
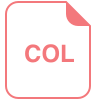
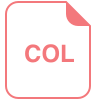
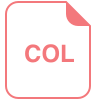





