请修改上述回答,其中按键1可以读取一个文件路径,按键2需要获取到这个文件路径
时间: 2023-06-14 15:06:51 浏览: 39
好的,以下是修改后的回答:
抱歉,我的回答有点误导。我之前提到的 ChitGPT 是一个由 CSDN 开发的类 ChatGPT 的 AI,而与之类似的 ChatGPT 模型由 OpenAI 公司开发。这两个模型都采用了类似的技术,但是它们是由不同的团队开发的,因此它们之间没有直接的关联。
关于您的问题,如果您想让按键1读取一个文件路径,您需要编写一个函数来实现这个功能。例如,您可以使用 Python 的 Tkinter 模块来创建一个简单的 GUI,然后在该 GUI 中添加一个按钮,使其在单击时打开一个文件对话框,以便用户选择文件并将其路径存储到变量中。然后,您可以使用该变量来执行其他操作,例如在按键2中使用该路径来读取文件内容。
相关问题
编写matlab app designer 脚本,其中按键1可以读取一个文件路径,按键2需要获取到这个文件路径
下面是一个简单的示例代码,可以实现你所需要的功能:
```matlab
classdef MyApp < matlab.apps.AppBase
% Properties
properties (Access = private)
FilePath % 文件路径
end
% UI组件
properties (Access = public)
UIFigure matlab.ui.Figure
Button1 matlab.ui.control.Button
Button2 matlab.ui.control.Button
FilePathLabel matlab.ui.control.Label
end
% Methods
methods (Access = private)
% 按钮1点击事件处理函数
function onButton1Pushed(app, event)
[file,path] = uigetfile('*.*');
if isequal(file,0)
return;
end
app.FilePath = fullfile(path, file);
app.FilePathLabel.Text = app.FilePath;
end
% 按钮2点击事件处理函数
function onButton2Pushed(app, event)
if isempty(app.FilePath)
app.FilePathLabel.Text = '请先选择文件';
else
disp(app.FilePath);
end
end
end
% App初始化函数
methods (Access = private)
% 创建UI组件
function createComponents(app)
% 创建主窗口
app.UIFigure = uifigure('Name', 'My App', 'Position', [100 100 300 150]);
% 创建按钮1
app.Button1 = uibutton(app.UIFigure, 'push', 'Position', [30 70 100 22], 'Text', '选择文件', 'ButtonPushedFcn', @(btn,event)onButton1Pushed(app,event));
% 创建按钮2
app.Button2 = uibutton(app.UIFigure, 'push', 'Position', [170 70 100 22], 'Text', '获取文件路径', 'ButtonPushedFcn', @(btn,event)onButton2Pushed(app,event));
% 创建文件路径标签
app.FilePathLabel = uilabel(app.UIFigure, 'Position', [30 30 240 22], 'Text', '');
end
end
% App creation and deletion
methods (Access = public)
% 创建App
function app = MyApp
% 创建UI组件
createComponents(app);
% 指定App名称
app.UIFigure.Name = 'My App';
% 初始化文件路径为空
app.FilePath = '';
end
% 删除App
function delete(app)
% 关闭主窗口
delete(app.UIFigure);
end
end
end
```
使用方法:
1. 将上述代码保存为 `MyApp.m` 文件;
2. 在 Matlab 命令窗口中输入 `app = MyApp` 来运行 App;
3. 点击按钮1选择一个文件;
4. 点击按钮2获取文件路径。
其中,文件路径会存储在 `app.FilePath` 属性中,可以在其他函数中使用。在本示例中,我们在按钮2的点击事件处理函数中使用了 `disp(app.FilePath)` 来将文件路径打印到命令窗口中。同时,我们也在主窗口中添加了一个标签,用来显示文件路径。
使用按键精灵读取一个txt文档内容并复制到剪贴板
好的,我可以为您提供一个使用按键精灵读取一个txt文档内容并复制到剪贴板的示例脚本:
```
// 打开txt文档
ShellExecute(0, "open", "notepad.exe", "D:\\test.txt", "", SW_SHOW);
// 等待notepad打开并获取焦点
Delay(1000);
SetWindowState(GetWindowState("无标题 - 记事本"), WINDOW_RESTORE);
SetWindowState(GetWindowState("无标题 - 记事本"), WINDOW_FOCUS);
// 全选并复制文本
KeyDown(VK_CONTROL);
KeyPress('A');
KeyUp(VK_CONTROL);
KeyDown(VK_CONTROL);
KeyPress('C');
KeyUp(VK_CONTROL);
// 获取剪贴板内容并输出
var content = GetClipboard();
alert(content);
```
请注意,这个脚本假设您的txt文档路径是`D:\test.txt`,您需要根据实际情况修改路径。同时,您也可以根据需要修改等待时间和窗口标题等参数。
相关推荐
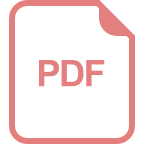
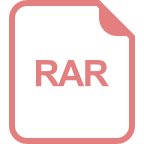
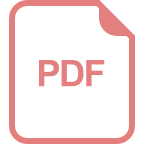












