用C语言编程实现使用EGE库设计一个绘制多边形的程序。并且满足以下要求: 1、可以绘制任意个多边形; 2、过程中要显示绘制路径,绘制结束后,填充多边形内部; 3、能够将已绘制完成的多边形保存到文件中,并且可以从文件中读取已保存的多边形,重新显示在界面上。 4、用鼠标左键绘制多边形 5、用鼠标右击结束绘制 6、用键盘“S”将多边形保存到文件中 7、用键盘“L”将多边形从文件中读出
时间: 2024-04-04 12:34:01 浏览: 69
好的,以下是实现该要求的C语言代码:
```
#include <graphics.h>
#include <stdio.h>
#define MAX_POLYGONS 10 // 最多绘制多边形个数
#define MAX_POINTS 100 // 每个多边形最多顶点数
struct Polygon {
int points[MAX_POINTS][2]; // 多边形顶点坐标
int num_points; // 多边形顶点数
};
int main() {
int gd = DETECT, gm, i, j;
initgraph(&gd, &gm, "");
setcolor(WHITE);
struct Polygon polygons[MAX_POLYGONS];
int num_polygons = 0;
int drawing = 0;
int current_polygon = 0;
while (1) {
if (kbhit()) { // 按键事件处理
char ch = getch();
if (ch == 's' || ch == 'S') { // 保存多边形到文件
char filename[20];
printf("Enter filename: ");
scanf("%s", filename);
FILE *fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to open file!\n");
continue;
}
fprintf(fp, "%d\n", num_polygons);
for (i = 0; i < num_polygons; i++) {
fprintf(fp, "%d\n", polygons[i].num_points);
for (j = 0; j < polygons[i].num_points; j++) {
fprintf(fp, "%d %d\n", polygons[i].points[j][0], polygons[i].points[j][1]);
}
}
fclose(fp);
printf("Polygon saved to %s.\n", filename);
} else if (ch == 'l' || ch == 'L') { // 从文件读入多边形
char filename[20];
printf("Enter filename: ");
scanf("%s", filename);
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file!\n");
continue;
}
fscanf(fp, "%d", &num_polygons);
for (i = 0; i < num_polygons; i++) {
fscanf(fp, "%d", &polygons[i].num_points);
for (j = 0; j < polygons[i].num_points; j++) {
fscanf(fp, "%d %d", &polygons[i].points[j][0], &polygons[i].points[j][1]);
}
}
fclose(fp);
printf("Polygon loaded from %s.\n", filename);
}
}
if (ismouseclick(WM_LBUTTONDOWN)) { // 鼠标左键按下
if (!drawing) { // 开始绘制多边形
current_polygon = num_polygons;
num_polygons++;
drawing = 1;
polygons[current_polygon].num_points = 0;
}
int x = mousex();
int y = mousey();
if (polygons[current_polygon].num_points > 0) { // 绘制多边形边界
line(polygons[current_polygon].points[polygons[current_polygon].num_points - 1][0],
polygons[current_polygon].points[polygons[current_polygon].num_points - 1][1], x, y);
}
polygons[current_polygon].points[polygons[current_polygon].num_points][0] = x; // 记录顶点坐标
polygons[current_polygon].points[polygons[current_polygon].num_points][1] = y;
polygons[current_polygon].num_points++;
}
if (ismouseclick(WM_RBUTTONDOWN)) { // 鼠标右键按下
if (drawing) { // 结束绘制多边形
drawing = 0;
setfillstyle(SOLID_FILL, WHITE);
fillpoly(polygons[current_polygon].num_points, &polygons[current_polygon].points[0][0]); // 填充多边形
}
}
clearmouseclick(WM_LBUTTONDOWN);
clearmouseclick(WM_RBUTTONDOWN);
}
closegraph();
return 0;
}
```
程序中使用了一个结构体`Polygon`来表示一个多边形,其中包括一个二维数组`points`用来存储多边形的顶点坐标,一个整数`num_points`表示顶点数。
程序中使用`num_polygons`和`current_polygon`来记录当前已绘制多少个多边形和正在绘制的多边形的索引,使用`drawing`来记录当前是否正在绘制多边形。
程序中使用了`ismouseclick`函数来检测鼠标事件,使用`mousex`和`mousey`函数来获取鼠标的位置。
在绘制多边形过程中,每当鼠标左键按下时,程序会记录鼠标的位置,如果当前正在绘制多边形,则将当前点与上一个点之间连一条线段,并将当前点存入多边形的顶点数组中。
当鼠标右键按下时,如果当前正在绘制多边形,则将`drawing`设为0,表示绘制结束,使用`fillpoly`函数填充多边形内部。
在按下`s`或`S`键时,程序会提示用户输入文件名,将多边形数据保存到文件中;在按下`l`或`L`键时,程序会提示用户输入文件名,从文件中读入多边形数据。
注意:由于EGE库不支持UTF-8编码,请确认代码保存为ANSI编码。
阅读全文
相关推荐
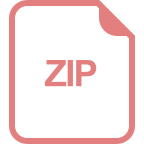










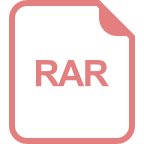
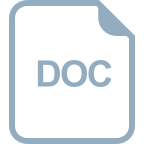



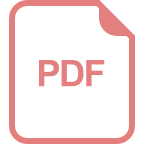