用web前端写一个推箱子,最少三个箱子
时间: 2023-07-14 13:14:31 浏览: 81
推箱子是一种经典的益智游戏,可以用web前端技术来实现。以下是一个简单的推箱子游戏的实现:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>推箱子</title>
<style>
body {
margin: 0;
padding: 0;
}
#game-board {
display: flex;
flex-wrap: wrap;
width: 400px;
margin: 30px auto;
border: 1px solid #ccc;
}
.cell {
width: 50px;
height: 50px;
border: 1px solid #ccc;
box-sizing: border-box;
background-color: #fff;
text-align: center;
line-height: 50px;
font-size: 24px;
}
.wall {
background-color: #000;
}
.player {
background-color: #f00;
}
.box {
background-color: #00f;
}
.target {
background-color: #0f0;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script src="main.js"></script>
</body>
</html>
```
CSS样式:
- `body`元素的`margin`和`padding`都设置为0,以去除默认的外边距和内边距。
- `#game-board`元素使用`flex`布局,设置了宽度、边距和边框样式。
- `.cell`元素设置了宽度、高度、边框样式、背景颜色、文本居中、字体大小和行高。
- `.wall`、`.player`、`.box`和`.target`元素分别表示墙、玩家、箱子和目标点的样式。
JavaScript代码:
```javascript
// 地图数据
const mapData = [
'##########',
'# #',
'# #',
'# # @ #',
'# #### #',
'# # #',
'# # * #',
'# # #',
'# #',
'##########'
];
// 地图对象
const map = {
width: 0,
height: 0,
cells: []
};
// 玩家位置
const playerPos = {
x: 0,
y: 0
};
// 箱子位置
const boxPos = {
x: 0,
y: 0
};
// 初始化地图
function initMap() {
const gameBoardEl = document.getElementById('game-board');
map.width = mapData[0].length;
map.height = mapData.length;
for (let y = 0; y < map.height; y++) {
const row = [];
for (let x = 0; x < map.width; x++) {
const cell = document.createElement('div');
cell.classList.add('cell');
switch (mapData[y][x]) {
case '#':
cell.classList.add('wall');
break;
case '@':
cell.classList.add('player');
playerPos.x = x;
playerPos.y = y;
break;
case '*':
cell.classList.add('box');
boxPos.x = x;
boxPos.y = y;
break;
case ' ':
break;
default:
cell.classList.add('target');
break;
}
row.push(cell);
gameBoardEl.appendChild(cell);
}
map.cells.push(row);
}
}
// 移动玩家
function movePlayer(dx, dy) {
const x = playerPos.x + dx;
const y = playerPos.y + dy;
if (x < 0 || x >= map.width || y < 0 || y >= map.height) {
return;
}
const cell = map.cells[y][x];
if (cell.classList.contains('wall')) {
return;
}
if (cell.classList.contains('box')) {
const bx = boxPos.x + dx;
const by = boxPos.y + dy;
if (bx < 0 || bx >= map.width || by < 0 || by >= map.height) {
return;
}
const boxCell = map.cells[by][bx];
if (boxCell.classList.contains('wall') || boxCell.classList.contains('box')) {
return;
}
boxCell.classList.add('box');
cell.classList.remove('box');
boxPos.x = bx;
boxPos.y = by;
}
playerPos.x = x;
playerPos.y = y;
map.cells[playerPos.y][playerPos.x].classList.add('player');
cell.classList.remove('player');
checkWin();
}
// 检查是否胜利
function checkWin() {
const targets = document.querySelectorAll('.target');
const boxes = document.querySelectorAll('.box');
let win = true;
for (let i = 0; i < targets.length; i++) {
if (!boxes[i] || targets[i] !== boxes[i].parentNode) {
win = false;
break;
}
}
if (win) {
alert('You win!');
}
}
// 监听按键事件
document.addEventListener('keydown', function(event) {
switch (event.keyCode) {
case 37: // 左
movePlayer(-1, 0);
break;
case 38: // 上
movePlayer(0, -1);
break;
case 39: // 右
movePlayer(1, 0);
break;
case 40: // 下
movePlayer(0, 1);
break;
}
});
// 初始化
initMap();
```
JavaScript代码包含以下几个部分:
- `mapData`数组表示地图的数据,其中`#`表示墙,`@`表示玩家,`*`表示箱子,空格表示空地,其他字符表示目标点。
- `map`对象表示地图的状态,包括宽度、高度和每个单元格的状态。
- `playerPos`对象表示玩家的位置。
- `boxPos`对象表示箱子的位置。
- `initMap`函数根据`mapData`数组初始化地图,将每个单元格的状态保存到`map`对象中。
- `movePlayer`函数根据玩家的移动方向,更新玩家和箱子的位置,并更新地图的状态。
- `checkWin`函数检查是否胜利,即所有箱子是否都移动到了目标点上。
- `document.addEventListener`监听按键事件,根据按键更新玩家的位置。
在浏览器中打开HTML文件即可开始游戏。
相关推荐
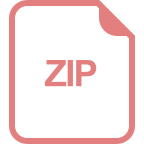












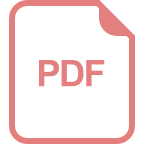