C++实现单链表的插入删除
时间: 2024-02-27 16:58:47 浏览: 66
单链表的插入删除可以通过以下代码实现:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
// 在链表头插入节点
ListNode* insertAtHead(ListNode* head, int val) {
ListNode* node = new ListNode(val);
node->next = head;
return node;
}
// 在链表尾插入节点
ListNode* insertAtTail(ListNode* head, int val) {
ListNode* node = new ListNode(val);
if (head == NULL) {
head = node;
} else {
ListNode* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = node;
}
return head;
}
// 在链表中删除节点
ListNode* deleteNode(ListNode* head, int val) {
if (head == NULL) {
return NULL;
} else if (head->val == val) {
ListNode* newHead = head->next;
delete head;
return newHead;
} else {
ListNode* cur = head;
while (cur->next != NULL && cur->next->val != val) {
cur = cur->next;
}
if (cur->next != NULL) {
ListNode* temp = cur->next;
cur->next = cur->next->next;
delete temp;
}
return head;
}
}
// 打印链表
void printList(ListNode* head) {
ListNode* cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
ListNode* head = NULL;
// 在链表头插入节点
head = insertAtHead(head, 1);
head = insertAtHead(head, 2);
head = insertAtHead(head, 3);
printList(head); // 3 2 1
// 在链表尾插入节点
head = insertAtTail(head, 4);
head = insertAtTail(head, 5);
head = insertAtTail(head, 6);
printList(head); // 3 2 1 4 5 6
// 在链表中删除节点
head = deleteNode(head, 3);
head = deleteNode(head, 6);
printList(head); // 2 1 4 5
return 0;
}
```
阅读全文
相关推荐
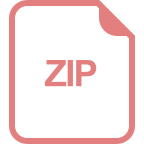
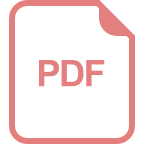
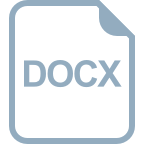















