c++扫雷程序完整代码
时间: 2023-12-23 13:02:23 浏览: 83
以下是一个简单的C++扫雷程序的完整代码:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <vector>
#include <string>
using namespace std;
// 定义扫雷方格的状态
enum SquareState {
UNREVEALED, // 未翻开
REVEALED, // 已翻开
FLAGGED // 已标记为地雷
};
// 定义扫雷方格
struct Square {
bool is_mine; // 是否是地雷
SquareState state; // 方格状态
int neighboring_mines; // 相邻的地雷数量
};
// 定义扫雷游戏
class Minesweeper {
private:
int rows; // 行数
int cols; // 列数
int mines; // 地雷数量
vector<vector<Square>> board; // 扫雷棋盘
bool game_over; // 游戏结束标志
// 随机生成地雷位置
void generate_mines() {
int count = 0;
while (count < mines) {
int row = rand() % rows;
int col = rand() % cols;
if (!board[row][col].is_mine) {
board[row][col].is_mine = true;
count++;
}
}
}
// 计算每个方格周围的地雷数量
void count_neighboring_mines() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (!board[i][j].is_mine) {
int count = 0;
for (int k = i - 1; k <= i + 1; k++) {
for (int l = j - 1; l <= j + 1; l++) {
if (k >= 0 && k < rows && l >= 0 && l < cols && board[k][l].is_mine) {
count++;
}
}
}
board[i][j].neighboring_mines = count;
}
}
}
}
// 翻开指定方格
void reveal_square(int row, int col) {
if (board[row][col].state == UNREVEALED) {
board[row][col].state = REVEALED;
if (board[row][col].is_mine) {
game_over = true;
}
else if (board[row][col].neighboring_mines == 0) {
for (int i = row - 1; i <= row + 1; i++) {
for (int j = col - 1; j <= col + 1; j++) {
if (i >= 0 && i < rows && j >= 0 && j < cols && !(i == row && j == col)) {
reveal_square(i, j);
}
}
}
}
}
}
public:
// 构造函数
Minesweeper(int r, int c, int m) : rows(r), cols(c), mines(m), game_over(false) {
// 初始化棋盘
board.resize(rows);
for (int i = 0; i < rows; i++) {
board[i].resize(cols);
for (int j = 0; j < cols; j++) {
board[i][j].is_mine = false;
board[i][j].state = UNREVEALED;
board[i][j].neighboring_mines = 0;
}
}
// 随机生成地雷位置
srand(time(NULL));
generate_mines();
// 计算每个方格周围的地雷数量
count_neighboring_mines();
}
// 显示棋盘
void show_board() {
cout << " ";
for (int j = 0; j < cols; j++) {
cout << j << " ";
}
cout << endl;
cout << " ";
for (int j = 0; j < cols; j++) {
cout << "--";
}
cout << endl;
for (int i = 0; i < rows; i++) {
cout << i << " | ";
for (int j = 0; j < cols; j++) {
if (game_over && board[i][j].is_mine) {
cout << "* ";
}
else if (board[i][j].state == UNREVEALED) {
cout << ". ";
}
else if (board[i][j].state == FLAGGED) {
cout << "F ";
}
else {
cout << board[i][j].neighboring_mines << " ";
}
}
cout << endl;
}
}
// 翻开指定方格
void play(int row, int col) {
if (game_over) {
cout << "Game over." << endl;
return;
}
if (board[row][col].state == REVEALED) {
cout << "This square has already been revealed." << endl;
return;
}
if (board[row][col].state == FLAGGED) {
cout << "This square has been flagged." << endl;
return;
}
reveal_square(row, col);
if (game_over) {
cout << "Game over." << endl;
}
}
// 标记指定方格为地雷
void flag(int row, int col) {
if (game_over) {
cout << "Game over." << endl;
return;
}
if (board[row][col].state == REVEALED) {
cout << "This square has already been revealed." << endl;
return;
}
if (board[row][col].state == FLAGGED) {
board[row][col].state = UNREVEALED;
}
else {
board[row][col].state = FLAGGED;
}
}
// 判断游戏是否已结束
bool is_game_over() {
return game_over;
}
};
// 主函数
int main() {
int rows, cols, mines;
cout << "Enter the number of rows: ";
cin >> rows;
cout << "Enter the number of columns: ";
cin >> cols;
cout << "Enter the number of mines: ";
cin >> mines;
Minesweeper game(rows, cols, mines);
game.show_board();
while (!game.is_game_over()) {
string action;
int row, col;
cout << "Enter an action (play/flag): ";
cin >> action;
cout << "Enter the row and column numbers: ";
cin >> row >> col;
if (action == "play") {
game.play(row, col);
}
else if (action == "flag") {
game.flag(row, col);
}
game.show_board();
}
return 0;
}
```
这个程序实现了以下功能:
1. 随机生成地雷位置;
2. 计算每个方格周围的地雷数量;
3. 翻开指定方格;
4. 标记指定方格为地雷;
5. 显示扫雷棋盘;
6. 判断游戏是否已结束。
可以通过在命令行中输入行数、列数和地雷数量来启动游戏,并通过输入“play”或“flag”来进行操作。在游戏过程中,程序会实时更新棋盘状态并提示游戏是否已结束。
阅读全文
相关推荐
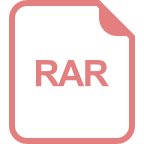
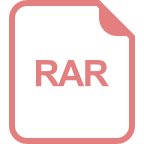

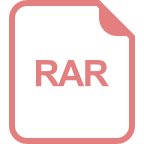
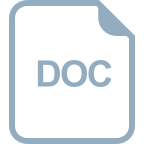
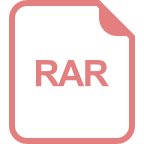





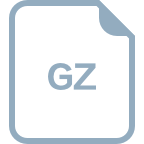