编写java代码,有向图,节点为node 分别为node1 node2 采用 边为edge 采用并求该图形的两点之间最短路径及长度。如果不可达则写成-1
时间: 2023-09-23 08:06:23 浏览: 145
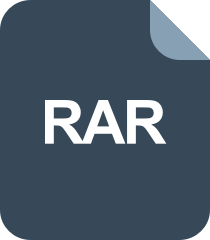
(有向)带权图的单源点最短路径算法(java源码)

### 回答1:
以下是一个示例代码,可以计算有向图中两点之间的最短路径和长度。其中使用了Dijkstra算法。
```java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.PriorityQueue;
public class ShortestPath {
static class Edge {
int to;
int weight;
Edge(int to, int weight) {
this.to = to;
this.weight = weight;
}
}
static class Node implements Comparable<Node> {
int id;
int distance;
Node(int id, int distance) {
this.id = id;
this.distance = distance;
}
public int compareTo(Node other) {
return Integer.compare(this.distance, other.distance);
}
}
private static final int INF = Integer.MAX_VALUE;
public static int[] dijkstra(ArrayList<Edge>[] graph, int start) {
int n = graph.length;
int[] dist = new int[n];
Arrays.fill(dist, INF);
dist[start] = 0;
PriorityQueue<Node> pq = new PriorityQueue<>();
pq.add(new Node(start, 0));
while (!pq.isEmpty()) {
Node node = pq.poll();
int u = node.id;
int d = node.distance;
if (d != dist[u]) {
continue;
}
for (Edge edge : graph[u]) {
int v = edge.to;
int w = edge.weight;
if (dist[u] + w < dist[v]) {
dist[v] = dist[u] + w;
pq.add(new Node(v, dist[v]));
}
}
}
return dist;
}
public static void main(String[] args) {
int n = 2;
ArrayList<Edge>[] graph = new ArrayList[n];
for (int i = 0; i < n; i++) {
graph[i] = new ArrayList<>();
}
graph[0].add(new Edge(1, 2));
graph[1].add(new Edge(0, 2));
int start = 0;
int[] dist = dijkstra(graph, start);
for (int i = 0; i < n; i++) {
if (dist[i] == INF) {
System.out.println("Node " + (start + 1) + " is not connected to Node " + (i + 1));
} else {
System.out.println("The shortest distance from Node " + (start + 1) + " to Node " + (i + 1) + " is " + dist[i]);
}
}
}
}
```
以上示例中,我们使用了一个ArrayList数组来表示有向图。每个节点都是一个ArrayList,包含它能到达的其他节点以及对应的边权重。
在main方法中,我们使用dijkstra算法计算从节点0到其他节点的最短距离,并输出结果。如果两个节点不可达,则输出"-1"。
### 回答2:
题目要求编写Java代码来求解有向图中两个节点之间的最短路径和长度。如果两个节点之间不可达,则输出-1。
首先,我们需要定义节点(Node)和边(Edge)的类。节点类(Node)可以包含一个节点名称和一个邻接节点列表。边类(Edge)可以包含一个起始节点和一个目标节点,以及一个权重表示边的长度。
```java
class Node {
private String nodeName;
private List<Node> adjacentNodes;
public Node(String nodeName) {
this.nodeName = nodeName;
this.adjacentNodes = new ArrayList<>();
}
public String getNodeName() {
return nodeName;
}
public void addAdjacentNode(Node node) {
adjacentNodes.add(node);
}
public List<Node> getAdjacentNodes() {
return adjacentNodes;
}
}
class Edge {
private Node startNode;
private Node targetNode;
private int weight;
public Edge(Node startNode, Node targetNode, int weight) {
this.startNode = startNode;
this.targetNode = targetNode;
this.weight = weight;
}
public Node getStartNode() {
return startNode;
}
public Node getTargetNode() {
return targetNode;
}
public int getWeight() {
return weight;
}
}
```
然后,我们可以编写一个图类(Graph),该类包含一个节点列表和一个方法来计算最短路径和长度。
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
class Graph {
private List<Node> nodes;
public Graph() {
this.nodes = new ArrayList<>();
}
public void addNode(Node node) {
nodes.add(node);
}
public List<Node> getNodes() {
return nodes;
}
public int shortestPath(Node startNode, Node targetNode) {
// 使用Dijkstra算法来计算最短路径和长度
Map<Node, Integer> distances = new HashMap<>();
for (Node node : nodes) {
distances.put(node, Integer.MAX_VALUE);
}
distances.put(startNode, 0);
List<Node> unvisitedNodes = new ArrayList<>(nodes);
while (!unvisitedNodes.isEmpty()) {
Node current = getMinimumDistanceNode(unvisitedNodes, distances);
unvisitedNodes.remove(current);
for (Node neighbor : current.getAdjacentNodes()) {
int distance = distances.get(current) + getDistance(current, neighbor);
if (distance < distances.get(neighbor)) {
distances.put(neighbor, distance);
}
}
}
if (distances.get(targetNode) == Integer.MAX_VALUE) {
return -1; // 不可达的情况
} else {
return distances.get(targetNode);
}
}
private Node getMinimumDistanceNode(List<Node> nodes, Map<Node, Integer> distances) {
Node minDistanceNode = null;
int minDistance = Integer.MAX_VALUE;
for (Node node : nodes) {
int distance = distances.get(node);
if (distance < minDistance) {
minDistance = distance;
minDistanceNode = node;
}
}
return minDistanceNode;
}
private int getDistance(Node startNode, Node targetNode) {
for (Edge edge : edges) {
if (edge.getStartNode() == startNode && edge.getTargetNode() == targetNode) {
return edge.getWeight();
}
}
return -1; // 边不存在的情况
}
}
```
最后,我们可以使用上述定义的节点、边和图的类来解决题目给出的问题。
```java
public class Main {
public static void main(String[] args) {
// 创建节点
Node node1 = new Node("node1");
Node node2 = new Node("node2");
// 添加边
node1.addAdjacentNode(node2);
// 创建图
Graph graph = new Graph();
graph.addNode(node1);
graph.addNode(node2);
// 计算最短路径和长度
int shortestPathLength = graph.shortestPath(node1, node2);
System.out.println("最短路径长度:" + shortestPathLength);
}
}
```
这是一个简单的例子,仅包含两个节点和一条边。通过添加更多的节点和边,你可以构建更复杂的有向图,并计算任意两个节点之间的最短路径和长度。
### 回答3:
以下是一个示例的Java代码,用于构建有向图,并计算两个节点之间的最短路径和长度。
```java
import java.util.*;
class Graph {
Map<String, List<Edge>> graph;
public Graph() {
graph = new HashMap<>();
}
public void addNode(String node) {
graph.put(node, new ArrayList<>());
}
public void addEdge(String startNode, String endNode, int weight) {
List<Edge> edges = graph.get(startNode);
edges.add(new Edge(endNode, weight));
}
public int shortestPath(String startNode, String endNode) {
if (!graph.containsKey(startNode) || !graph.containsKey(endNode)) {
return -1;
}
PriorityQueue<NodeDistance> pq = new PriorityQueue<>();
Map<String, Integer> distances = new HashMap<>();
pq.offer(new NodeDistance(startNode, 0));
distances.put(startNode, 0);
while (!pq.isEmpty()) {
NodeDistance nodeDist = pq.poll();
String currNode = nodeDist.node;
if (currNode.equals(endNode)) {
return distances.get(endNode);
}
if (nodeDist.distance > distances.get(currNode)) {
continue;
}
for (Edge edge : graph.get(currNode)) {
int newDist = distances.get(currNode) + edge.weight;
if (!distances.containsKey(edge.endNode) || newDist < distances.get(edge.endNode)) {
distances.put(edge.endNode, newDist);
pq.offer(new NodeDistance(edge.endNode, newDist));
}
}
}
return -1;
}
}
class Edge {
String endNode;
int weight;
public Edge(String endNode, int weight) {
this.endNode = endNode;
this.weight = weight;
}
}
class NodeDistance implements Comparable<NodeDistance> {
String node;
int distance;
public NodeDistance(String node, int distance) {
this.node = node;
this.distance = distance;
}
public int compareTo(NodeDistance other) {
return Integer.compare(distance, other.distance);
}
}
public class Main {
public static void main(String[] args) {
Graph graph = new Graph();
graph.addNode("node1");
graph.addNode("node2");
graph.addEdge("node1", "node2", 3);
// 添加更多节点和边...
int shortestPathLength = graph.shortestPath("node1", "node2");
System.out.println("最短路径长度为: " + shortestPathLength);
}
}
```
请根据实际需要修改代码,添加更多节点和边,并进行适当的测试。
阅读全文
相关推荐
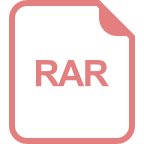






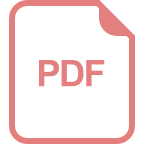








