#include <unistd.h> #include <sys/types.h> #include <sys/wait.h> #include <stdio.h> /************************ * 返回值: 调用成功且子进程正常退出返回退出代码,否则返回-1 *************************/ int waitProcess() { int status = -1; /********** BEGIN **********/ /********** END **********/ printf("子进程正常退出,退出代码:%d\n", WEXITSTATUS(status)); return status; }补全代码
时间: 2023-06-29 17:12:18 浏览: 61
这段代码中缺少的部分是调用wait函数等待子进程退出并获取其退出状态,可以使用如下代码进行补全:
```c
pid_t child_pid = wait(&status);
if (child_pid == -1) {
perror("wait failed");
return -1;
}
```
其中wait函数会阻塞等待子进程退出,并将退出状态保存到status变量中,返回值为子进程的PID。如果wait调用失败,则返回-1,并打印错误信息。
完整的waitProcess函数代码如下:
```c
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <stdio.h>
/************************
* 返回值: 调用成功且子进程正常退出返回退出代码,否则返回-1
*************************/
int waitProcess() {
int status = -1;
pid_t child_pid = wait(&status);
if (child_pid == -1) {
perror("wait failed");
return -1;
}
if (WIFEXITED(status)) {
printf("子进程正常退出,退出代码:%d\n", WEXITSTATUS(status));
} else if (WIFSIGNALED(status)) {
printf("子进程被信号终止,信号号码:%d\n", WTERMSIG(status));
} else if (WIFSTOPPED(status)) {
printf("子进程被信号停止,信号号码:%d\n", WSTOPSIG(status));
} else {
printf("子进程状态未知\n");
}
return status;
}
```
补全后的代码中增加了对子进程状态的判断,包括正常退出、被信号终止、被信号停止等情况,并打印相应的信息。
相关推荐
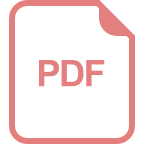
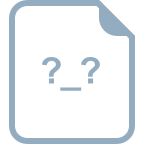
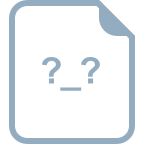
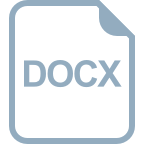
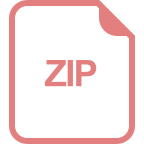
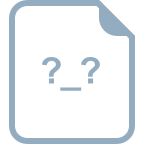
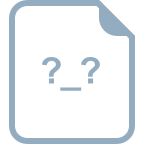
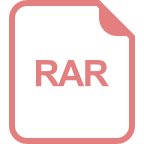
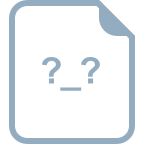
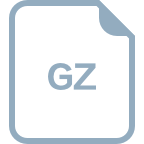
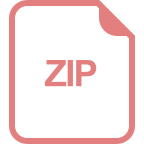