#include <sys/types.h> #include <stdio.h> #include <unistd.h> int main() { pid_t pid; /* fork a child process */ pid = fork(); if (pid < 0) { /* error occurred */ fprintf(stderr, "Fork Failed"); return 1; } else if (pid == 0) { /* 子进程 */ execlp("/bin/ls","ls",NULL); } else { /* 父进程 */ /* 父进程将一直等待,直到子进程运行完毕*/ wait(NULL); printf("Child Complete"); } return 0;
时间: 2024-03-11 10:46:22 浏览: 19
这段代码是一个简单的进程创建和等待子进程运行完毕的示例。在程序运行时,它会创建一个子进程,并在子进程中执行 `ls` 命令。父进程会等待子进程执行完毕后才会继续执行,并输出 "Child Complete"。
具体来说,这段代码中使用了 `fork()` 函数创建子进程。`fork()` 函数会返回两次,一次在父进程中返回子进程的 PID,另一次在子进程中返回 0,因此可以通过返回值来区分父进程和子进程的代码执行路径。
在子进程中,使用 `execlp()` 函数执行 `ls` 命令。`execlp()` 函数可以在当前进程中执行一个新程序,它接受三个参数:第一个参数是要执行的程序的路径,第二个参数是程序的名称,第三个参数是一个 NULL 指针,表示参数列表结束。
在父进程中,使用 `wait()` 函数等待子进程执行完毕。`wait()` 函数会一直阻塞父进程,直到任意一个子进程退出。在这个例子中,父进程传入了一个 NULL 参数,表示不关心子进程的退出状态。最后,父进程输出 "Child Complete",程序结束。
相关问题
例1. 编写如下程序,保存为forkt1.c。 #include <sys/types.h> #include <unistd.h> #include <stdio.h> void main() {
这是一个使用fork函数创建子进程的C程序。程序的主要功能是创建一个子进程,并在子进程中输出一条信息。
代码如下:
```c
#include <sys/types.h>
#include <unistd.h>
#include <stdio.h>
int main()
{
pid_t pid;
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork failed\n");
return 1;
} else if (pid == 0) {
printf("This is the child process\n");
} else {
printf("This is the parent process\n");
}
return 0;
}
```
这个程序首先包含了一些头文件,然后定义了一个主函数`main()`。在`main()`函数中,首先声明了一个`pid_t`类型的变量`pid`,用于保存fork函数的返回值。
接下来,调用了fork函数,将返回值赋给`pid`变量。fork函数会创建一个新的进程,如果创建成功,则在父进程中返回子进程的PID,在子进程中返回0。如果创建失败,则返回-1。
然后,通过判断pid的值,可以确定当前是在父进程还是子进程中。如果pid小于0,则说明fork失败,输出错误信息。如果pid等于0,则说明当前是在子进程中,输出一条信息"This is the child process"。如果pid大于0,则说明当前是在父进程中,输出一条信息"This is the parent process"。
最后,主函数返回0,表示程序正常结束。
#include <stdio.h> #include <sys/types.h> #include <unistd.h> int main(){ pid_t a; a=fork(); printf("Tonly is coming ---%d\n",a); printf("1111\n"); while(1){ } return 0; }
这段代码可以正常编译运行,其作用是创建一个子进程,并在父子进程中分别输出当前进程的 ID(父进程输出父进程 ID,子进程输出子进程 ID),然后进入一个死循环。
需要注意的是,父进程和子进程是并发执行的,因此无法确定它们的输出顺序。在一些系统中,子进程的输出可能会先于父进程的输出,因此输出结果可能会与我们的预期不同。
如果想要让父进程等待子进程执行完毕后再继续执行,可以使用 `wait` 函数。例如:
```
#include <stdio.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <unistd.h>
int main(){
pid_t pid;
pid = fork();
if (pid == 0){ // 子进程
printf("I'm child process, my pid is %d\n", getpid());
// 子进程执行完毕后退出
return 0;
} else if (pid > 0){ // 父进程
printf("I'm parent process, my pid is %d\n", getpid());
// 父进程等待子进程执行完毕
wait(NULL);
printf("Child process has exited\n");
} else { // fork 出错
perror("fork");
return 1;
}
return 0;
}
```
该程序会先输出父进程的 ID,然后创建一个子进程,子进程输出自己的 ID 后退出,父进程等待子进程执行完毕后输出一条信息。
相关推荐
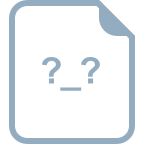
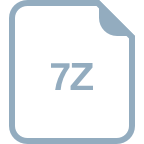
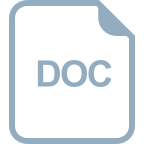













