> F<-function(x,n,m=NULL){ + + if(is.null(m)){ + y<-array(0,dim=c(n,m)) + m<-length(x)/n + for(i in (1:n)){ + t<-(i-1)*m-1 + k<-i + for(j in (1:m)){ + y[k,j]<-x[t+j-1] + + } + } + } + return(y) + } > F(1:12,4) Error in y[k, j] <- x[t + j - 1] : 矩阵里的下标数目不对
时间: 2023-06-26 12:10:25 浏览: 54
这个错误可能是因为在函数中定义了 `dim=c(n,m)`,但是在调用函数时只传入了一个参数 `n`。因此,`m` 的值为 `NULL`,导致在循环中 `m` 不能被正确地使用。要解决这个问题,可以在调用函数时同时传入 `n` 和 `m` 两个参数,或者在函数中使用默认参数来避免这个问题。以下是一个示例函数:
```
F <- function(x, n, m = NULL) {
if (is.null(m)) {
m <- length(x) / n
}
y <- matrix(0, nrow = n, ncol = m)
for (i in 1:n) {
t <- (i - 1) * m
for (j in 1:m) {
y[i, j] <- x[t + j]
}
}
return(y)
}
```
这个函数中使用了默认参数 `m = NULL`,如果在调用函数时不传入 `m` 参数,就使用 `length(x) / n` 计算 `m` 的值。另外,使用 `matrix()` 函数来创建一个空矩阵 `y`,并且在循环中修改 `t` 的计算方式。
相关问题
F<-function(x,n,m=NULL){ y<-0 if(m==NULL){ m<-length(x)/n for(i in (1:n)){ t<-i for(j in (1:m)){ y[t,j]<-x[t*j] y } } } } F(1:12,3)
这段代码存在一些问题,可能是因为复制时出现了错误。以下是我猜测的意思和可能的修复方法:
1. 函数定义中缺少返回语句。可以在函数末尾添加 `return(y)` 语句,以返回结果。
2. 在 for 循环中调用 y 变量,但 y 变量未定义。可以在函数开头添加 `y<-matrix(0,n,m)` 语句,以定义 y 变量并初始化为全零矩阵。
3. 在 for 循环中,t 变量未被更新。可以将 `t<-i` 改为 `t<-(i-1)*m+1`,以计算每列的起始索引。
4. 在 for 循环中,y 矩阵未被正确地填充。可以将 `y t,j<-x t*j` 改为 `y[i,j]<-x[t+j-1]`,以将 x 中对应位置的元素填充到 y 中。
综上所述,修复后的代码如下:
```
F<-function(x,n,m=NULL){
y<-matrix(0,n,m)
if(is.null(m)){
m<-length(x)/n
for(i in 1:n){
t<-(i-1)*m+1
for(j in 1:m){
y[i,j]<-x[t+j-1]
}
}
}
return(y)
}
F(1:12,3)
```
输出结果为:
```
[,1] [,2] [,3] [,4]
[1,] 1 4 7 10
[2,] 2 5 8 11
[3,] 3 6 9 12
```
这个函数的作用是将一个长度为 n*m 的向量 x 转化为一个 n 行、m 列的矩阵。如果调用时未指定 m,则默认为 length(x)/n。
F<-function(x,n,m=NULL){ if(is.null(m)){ y<-array(0,dim=c(n,m)) m<-length(x)/n for(i in (1:n)){ t<-(i-1)*m-1 k<-i for(j in (1:m)){ y[k,j]<-x[t+j-1] } } } return(y) } F(1:12,4)
这是一个 R 语言的函数,用于将一个一维向量转化为二维数组。其中,x 是需要转化的一维向量,n 是转化后的二维数组的行数,m 是转化后的二维数组的列数,如果不指定 m,则默认为 NULL。下面是该函数的具体实现:
1.首先判断是否指定了 m,如果没有,则计算出 m 的值为 length(x)/n。
2.创建一个全为 0 的二维数组 y,其行数为 n,列数为 m。
3.使用两个 for 循环将 x 中的元素逐个赋值给 y 中的元素,具体实现步骤如下:
(1)外层循环 i 遍历 y 的每一行;
(2)内层循环 j 遍历 y 的每一列;
(3)计算出当前元素在 x 中的下标 t=j+(i-1)*m;
(4)将 x[t] 的值赋给 y[i,j]。
4.返回转化后的二维数组 y。
在这里,函数调用 F(1:12,4) 将会返回一个 4 行 3 列的二维数组,其元素为从 1 到 12 的整数。
相关推荐
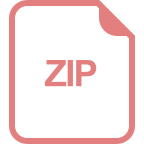
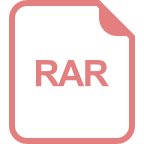
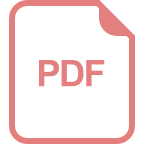











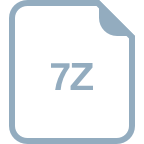
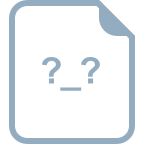