c++写一个程序,多个线程并行,其中一个线程完成后,其他线程也结束
时间: 2024-05-01 18:16:22 浏览: 92
这个问题可以使用线程同步机制来解决,比如使用信号量或互斥锁来控制线程的执行顺序。
以下是一个使用互斥锁来实现线程同步的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
pthread_mutex_t mutex;
int count = 0;
void *thread_func(void *arg) {
int id = *(int*)arg;
pthread_mutex_lock(&mutex);
printf("Thread %d started\n", id);
count++;
pthread_mutex_unlock(&mutex);
// 等待第一个线程完成
while (count < 1);
printf("Thread %d finished\n", id);
pthread_exit(NULL);
}
int main() {
int i;
pthread_t threads[5];
int thread_ids[5] = {1, 2, 3, 4, 5};
pthread_mutex_init(&mutex, NULL);
for (i = 0; i < 5; i++) {
pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
}
for (i = 0; i < 5; i++) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
return 0;
}
```
在这个程序中,我们创建了5个线程,并使用一个互斥锁来控制它们的执行顺序。当一个线程开始执行时,它会先获取互斥锁来保证自己是第一个执行的线程。然后它会增加计数器count的值,并释放互斥锁。其他线程也会执行相同的操作,但是它们会被阻塞在获取互斥锁这一步,等待第一个线程释放锁。
当第一个线程完成后,它会增加计数器的值,这样其他线程就不再被阻塞,可以继续执行。当它们完成后,程序就结束了。
阅读全文
相关推荐
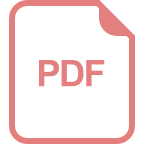
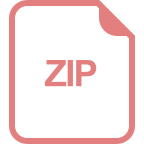
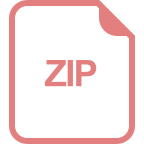
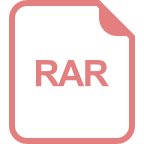
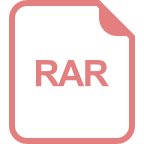
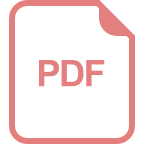
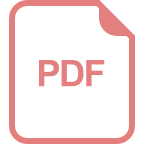
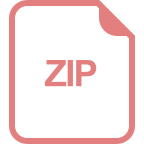
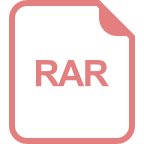
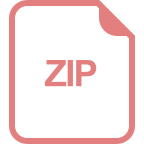
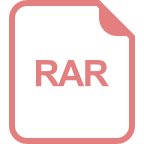
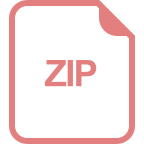
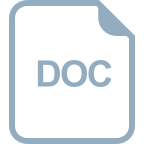
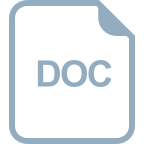
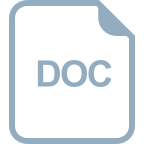
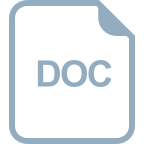