在java中使用list给定一个整数列表
时间: 2024-10-12 16:13:41 浏览: 29
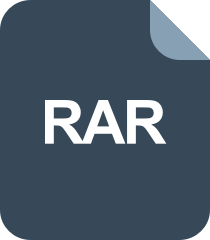
java求一个整数的因子.rar
在 Java 中,如果你有一个整数列表,你可以使用 ArrayList 或者 LinkedList 等 List 接口的实现来存储整数。这里是一个简单的示例:
```java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
// 创建一个ArrayList实例,用于存储整数
ArrayList<Integer> integerList = new ArrayList<>();
// 添加元素到列表中
integerList.add(10); // 添加第一个元素
integerList.add(20);
integerList.add(30);
// 访问列表元素
System.out.println("First element: " + integerList.get(0));
// 遍历列表
for (int i : integerList) {
System.out.print(i + " ");
}
// 判断是否包含特定元素
boolean containsTen = integerList.contains(10);
阅读全文
相关推荐
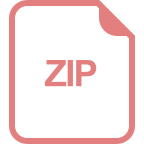
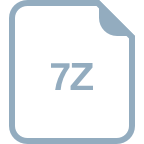















