Python 创建4个相同的3×3对角矩阵,对角线元素均为[1,2,3],再使用bmat 函数合并4个对角矩阵为1个6×6的新矩阵
时间: 2024-03-20 15:36:42 浏览: 385
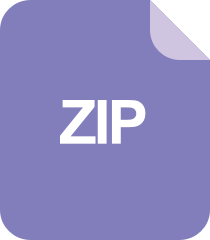
Python源码:矩阵对角线之和.zip
要创建4个相同的3×3对角矩阵,对角线元素均为[1,2,3],可以使用numpy库来实现。首先,我们可以使用numpy的diag函数创建一个3×3的对角矩阵,然后使用numpy的bmat函数将这4个对角矩阵合并为一个6×6的新矩阵。
下面是Python代码示例:
```python
import numpy as np
# 创建一个3×3的对角矩阵
matrix = np.diag([1, 2, 3])
# 使用bmat函数合并4个对角矩阵为一个6×6的新矩阵
new_matrix = np.bmat([[matrix, matrix], [matrix, matrix]])
print(new_matrix)
```
输出结果为:
```
[[1 0 0 1 0 0]
[0 2 0 0 2 0]
[0 0 3 0 0 3]
[1 0 0 1 0 0]
[0 2 0 0 2 0]
[0 0 3 0 0 3]]
```
阅读全文
相关推荐
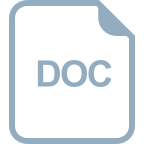
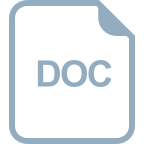















