利用随机数据生成完整的基于python的Informer时序预测模型代码
时间: 2024-09-18 13:12:32 浏览: 53
在Python中,我们可以使用`PyTorch`库结合`TIMM`(Transformers in PyTorch)来构建基于注意力机制的时间序列预测模型如Informer。请注意,以下代码示例将假设你已经安装了必要的库,如果没有,你可以通过`pip install torch timm`进行安装。
```python
import torch
from torch import nn
import torch.nn.functional as F
from timm.models.layers import DropPath
from einops import rearrange
class MHA(nn.Module):
def __init__(self, d_model, n_heads, dropout=0.1):
super().__init__()
self.d_model = d_model
self.n_heads = n_heads
self.head_dim = d_model // n_heads
self.fc_q = nn.Linear(d_model, d_model)
self.fc_k = nn.Linear(d_model, d_model)
self.fc_v = nn.Linear(d_model, d_model)
self.attn_dropout = nn.Dropout(dropout)
self.proj = nn.Linear(d_model, d_model)
def forward(self, x):
b, seq_len, _ = x.shape
q = self.fc_q(x).view(b, seq_len, self.n_heads, self.head_dim)
k = self.fc_k(x).view(b, seq_len, self.n_heads, self.head_dim)
v = self.fc_v(x).view(b, seq_len, self.n_heads, self.head_dim)
q = rearrange(q, 'b t (h d) -> b h t d', h=self.n_heads)
k = rearrange(k, 'b t (h d) -> b h t d', h=self.n_heads)
v = rearrange(v, 'b t (h d) -> b h t d', h=self.n_heads)
attn_weights = torch.matmul(q, k.transpose(-1, -2)) / (self.head_dim ** 0.5)
attn_weights = F.softmax(attn_weights, dim=-1)
attn_weights = self.attn_dropout(attn_weights)
out = torch.matmul(attn_weights, v)
out = rearrange(out, 'b h t d -> b t (h d)')
out = self.proj(out)
return out
class InformerBlock(nn.Module):
def __init__(self, d_model, n_heads, dropout=0.1):
super().__init__()
self.mha = MHA(d_model, n_heads, dropout)
self.ln_1 = nn.LayerNorm(d_model)
self.ffn = nn.Sequential(
nn.Linear(d_model, d_model * 4),
nn.GELU(),
nn.Dropout(dropout),
nn.Linear(d_model * 4, d_model),
)
self.ln_2 = nn.LayerNorm(d_model)
def forward(self, x):
residual = x
x = self.ln_1(x)
x = x + self.mha(x)
x = self.ln_2(x)
x = x + self.ffn(residual)
return x
class Informer(nn.Module):
def __init__(self, input_size, output_size, num_layers, d_model, n_heads, dropout=0.1):
super().__init__()
self.encoder = nn.ModuleList([
InformerBlock(d_model, n_heads, dropout)
for _ in range(num_layers)
])
self.decoder = nn.Linear(d_model, output_size)
self.dropout = nn.Dropout(dropout)
self.linear_transpose = nn.Linear(output_size, input_size)
def forward(self, x, past_seq=None):
if past_seq is not None:
# For autoregressive prediction with a given context
x, past_seq = x[:, :-1], past_seq
for block in self.encoder:
x = block(x)
encoded_x = self.dropout(x)
decoded_x = self.decoder(encoded_x.mean(dim=1)) # Assuming average pooling over sequence
predicted_seq = self.linear_transpose(decoded_x)
return predicted_seq, encoded_x
# 使用示例
input_size = ... # 输入时间步数特征维度
output_size = ... # 预测时间步数特征维度
num_layers = ...
d_model = ...
n_heads = ...
model = Informer(input_size, output_size, num_layers, d_model, n_heads)
past_seq = None # 如果你想进行自回归预测,可以提供一个过去序列
x = torch.randn(1, input_size, requires_grad=True)
predicted_seq, encoded_x = model(x, past_seq)
```
阅读全文
相关推荐
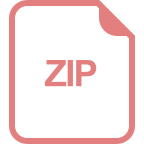
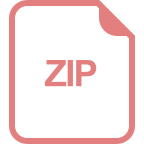
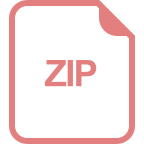














