#include <iostream> using namespace std; bool checkNumber(int num) { int square = num * num; return square % 1000 == num; } int main() { for (int num = 100; num < 1000; num++) { if (checkNumber(num)) { cout << num << " "; } } cout << endl; return 0; }能不能用for循环代替checkNumber
时间: 2024-04-12 20:28:15 浏览: 59
可以使用for循环代替checkNumber函数的逻辑。你可以直接在for循环中进行判断,而不需要额外定义一个函数。以下是使用for循环进行判断的修改后的代码:
```cpp
#include <iostream>
using namespace std;
int main() {
for (int num = 100; num < 1000; num++) {
int square = num * num;
if (square % 1000 == num) {
cout << num << " ";
}
}
cout << endl;
return 0;
}
```
这样修改后的代码与原来的代码逻辑相同,但省去了定义函数的步骤,直接在循环中进行判断。
相关问题
A factory produces products packed in square packets of the same height $h$ and of the sizes $1 \times 1, 2 \times 2, 3 \times 3, 4 \times 4, 5 \times 5, 6 \times 6$. These products are always delivered to customers in the square parcels of the same height $h$ as the products have and of the size $6 \times 6$. Because of the expenses it is the interest of the factory as well as of the customer to minimize the number of parcels necessary to deliver the ordered products from the factory to the customer. A good program solving the problem of finding the minimal number of parcels necessary to deliver the given products according to an order would save a lot of money. You are asked to make such a program. c++
torch.zeros((self.num_layers, batch_size, self.num_hiddens), device=self.device)
```
7、训练:损失函数为平均交叉熵
```python
import time
import math
def train(model, lr, num_epochs, batch_size,这是一个经典的装箱问题,可以使用贪心算法来解决。首先,我们将订单中每种 num_steps, use_random_iter=True):
loss_fn = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=产品的数量按照从大到小排序,然后依次将它们放入尽可能小的包裹中。具lr)
# 将模型放到device上
model.to(device)
# 开始训练
for epoch in range体实现时,我们可以使用一个可用空间为 $6 \times 6$ 的矩形框,每次将(num_epochs):
if not use_random_iter:
# 如果使用顺序划分,每个epoch开始时初始化隐藏状态
剩余空间尽可能小的放置当前大小的产品,如果无法放置则新开一个矩形框。以下 state = model.init_hidden(batch_size)
start_time = time.time()
l_sum, n = 0.0, 0是C++代码实现:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Product {
data_iter = get_batch(batch_size, num_steps, use_random_iter)
for X, Y in data_iter:
if use int size;
int count;
};
bool cmp(const Product& a, const Product& b) {
return a.count > b.count_random_iter:
# 如果使用随机采样,在每个小批量更新前初始化隐藏状态
state = model.init;
}
int minBoxes(vector<Product>& products) {
sort(products.begin(), products.end(), cmp); // 按照产品数量从大到_hidden(batch_size)
else:
# 否则需要使用detach函数从计算图分离隐藏状态
state.detach_()
小排序
int count = 0;
int boxSize = 6;
while (!products.empty()) {
int i = # 前向计算
outputs, state = model(X, state)
# 计算损失
y = Y.perm 0;
while (i < products.size()) {
if (products[i].count == 0) {
products.erase(products.beginute(1, 0, 2).reshape(-1, len(char_to_idx))
l = loss_fn(outputs, y.long())
() + i); // 移除数量为0的产品
} else if (boxSize >= products[i].size) {
box # 反向传播
optimizer.zero_grad()
l.backward()
# 梯度裁剪
grad_clipping(modelSize -= products[i].size;
products[i].count--;
} else {
break; // 空间不足,结束本次.parameters(), 1)
optimizer.step()
# 累加损失
l_sum += l.item() * y.shape[0]
放置
}
}
if (i == products.size()) {
count++; // 所有产品都已放置完毕 n += y.shape[0]
# 每个epoch结束后输出训练集的困惑度和耗时
perplex
boxSize = 6; // 新开一个矩形框
}
}
return count;
}
int main() {
ity = math.exp(l_sum / n)
print('epoch %d, perplexity %f, time %.2f sec' % ( vector<Product> products = {{1, 5}, {2, 3}, {3, 1}, {4, 2epoch + 1, perplexity, time.time() - start_time))
```
8、预测:给定一个前缀,进行}, {5, 4}, {6, 6}};
cout << minBoxes(products) << endl; // 输出4
return 0;
}
```
输入int类型范围内的n个非负整数,要求按各个整数的各数位上数字的平方和从小到大排序,若平方和相等则按数值从小到大排序。 例如,三个整数9、31、13各数位上数字的平方和分别为81、10、10,则排序结果为13、31、9。
### 回答1:
题目要求输入n个非负整数,按照各个整数的各数位上数字的平方和从小到大排序,如果平方和相等,则按数值从小到大排序。例如,输入9、31、13三个整数,它们的各数位上数字的平方和分别为81、10、10,则排序结果为13、31、9。
### 回答2:
这道题目的思路可以分成两步,第一步是对输入的每个整数分别求出各个数位上数字的平方和,第二步是按照平方和从小到大排序,若平方和相等则按照数值从小到大排序。
第一步可以使用一个函数来实现,输入一个整数,输出该整数各个数位上数字的平方和。我们可以循环求出每个数位上的数字,把数字的平方加起来。
第二步可以使用一个排序算法,比如快速排序(QuickSort)。排序的关键在于如何比较两个数的大小。我们可以先比较它们的平方和,如果平方和不同则直接根据数值大小进行比较;如果平方和相同则根据数值大小进行比较。排序的过程中,我们可以使用递归的方法对具有相同平方和的数字集合进行排序。
对于这道题目,我们可以使用一个结构体来表示输入的数字。结构体包含两个成员变量,一个是数字本身,另一个是各个数位上数字的平方和。我们可以先对每个数字进行一次遍历,计算出它的平方和,然后把它们存储在一个结构体数组中,最后对整个数组进行排序。排序之后,再遍历一次数组,输出其中的每个数字即可。
下面是代码示例:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
struct Number {
int value;
int square_sum;
};
int square_sum(int n) {
int sum = 0;
while (n) {
int digit = n % 10;
sum += digit * digit;
n /= 10;
}
return sum;
}
bool cmp(Number a, Number b) {
if (a.square_sum != b.square_sum) {
return a.square_sum < b.square_sum;
} else {
return a.value < b.value;
}
}
int main() {
int n;
cin >> n;
Number nums[n];
for (int i = 0; i < n; i++) {
int value;
cin >> value;
nums[i] = { value, square_sum(value) };
}
sort(nums, nums + n, cmp);
for (int i = 0; i < n; i++) {
cout << nums[i].value << endl;
}
return 0;
}
```
### 回答3:
本题要求按各个整数的各数位上数字的平方和从小到大排序,平方和相等则按数值从小到大排序,因此我们可以采用桶排序的思路进行求解。
首先,我们需要定义一个计算一个数各个数位上数字的平方和的函数,对于一个整数num,该函数可以使用如下的代码实现:
```
int getSquareSum(int num) {
int sum = 0;
while(num > 0) {
int digit = num % 10;
sum += digit * digit;
num /= 10;
}
return sum;
}
```
然后,我们可以遍历输入的n个非负整数,将它们放到对应的桶中,桶的编号是该整数各个数位上数字的平方和,即:
```
vector<int> buckets[810];
for(int i = 0; i < n; i++) {
int num;
cin >> num;
int squareSum = getSquareSum(num);
buckets[squareSum].push_back(num);
}
```
注意,由于各个整数的各数位上数字的平方和最大为729(即9的平方和乘以3),因此我们需要开一个大小为810的桶数组。
接下来,我们可以按照平方和从小到大的顺序遍历桶,对于每个桶中的数,我们都可以先将它们按数值从小到大排序,然后再输出即可,代码如下:
```
for(int i = 0; i < 810; i++) {
sort(buckets[i].begin(), buckets[i].end());
for(int j = 0; j < buckets[i].size(); j++) {
cout << buckets[i][j] << " ";
}
}
```
最后,整个程序的完整代码如下:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int getSquareSum(int num) {
int sum = 0;
while(num > 0) {
int digit = num % 10;
sum += digit * digit;
num /= 10;
}
return sum;
}
int main() {
int n;
cin >> n;
vector<int> buckets[810];
for(int i = 0; i < n; i++) {
int num;
cin >> num;
int squareSum = getSquareSum(num);
buckets[squareSum].push_back(num);
}
for(int i = 0; i < 810; i++) {
sort(buckets[i].begin(), buckets[i].end());
for(int j = 0; j < buckets[i].size(); j++) {
cout << buckets[i][j] << " ";
}
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
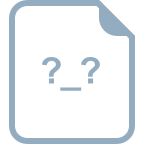





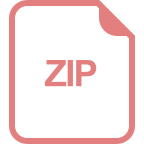
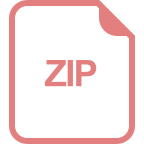