设计一个算法通过一趟遍历确定长度为n的单链表中值最大的结点
时间: 2024-10-25 08:09:54 浏览: 12
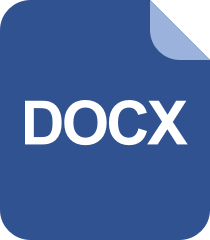
数据结构作业.docx
设计一个算法来找到长度为 n 的单链表中值最大的节点,可以采用迭代或递归的方式。这里我们选择迭代方法,因为它通常更高效,因为我们不需要额外的栈空间。
**步骤**:
1. 初始化两个变量,`current` 和 `maxNode`。将 `current` 设置为链表头节点,将 `maxNode` 设置为头节点(因为初始时所有节点都可能是最大值)。
2. 进行循环遍历:
- 比较当前节点的值 `current.value` 与 `maxNode.value`。如果 `current.value` 大于 `maxNode.value`,则更新 `maxNode` 为 `current`。
- 移动到下一个节点,即 `current = current.next`。
3. 当遍历完整个链表后,`maxNode` 就指向了链表中值最大的节点。
**伪代码**:
```python
def find_max_node(head):
if head is None:
return None
current = head
maxNode = current
while current.next is not None:
if current.value > maxNode.value:
maxNode = current
current = current.next
return maxNode
```
阅读全文
相关推荐
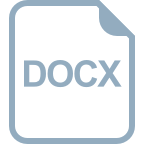
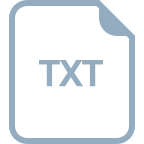















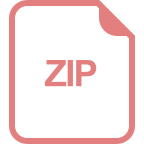
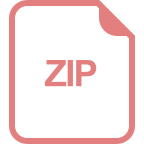