用c语言写一个代码线性表的链式存储实现单链表的插入、删除、查找、求表长、置逆以及有序单链表的合并算法的实现。
时间: 2024-09-23 22:07:53 浏览: 19
在C语言中,我们可以使用结构体来表示链表节点,并提供相应的函数来操作这个链表。这里是一个简单的单链表的实现,包括插入、删除、查找、求长度、反转和合并两个已排序链表的功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入节点到链表
Node* insert(Node* head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = head;
return newNode;
}
// 删除节点(按值删除)
Node* deleteNode(Node* head, int value) {
if (!head || !head->next) return head;
if (head->data == value) {
Node* temp = head;
head = head->next;
free(temp);
return head;
}
Node* current = head->next;
while (current && current->data != value) {
current = current->next;
}
if (!current) return head; // 没找到节点
Node* temp = current;
current = current->next;
free(temp);
return head;
}
// 查找节点
Node* search(Node* head, int value) {
Node* current = head;
while (current) {
if (current->data == value) return current;
current = current->next;
}
return NULL; // 如果找不到返回NULL
}
// 计算链表长度
int length(Node* head) {
int count = 0;
Node* current = head;
while (current) {
count++;
current = current->next;
}
return count;
}
// 反转链表
Node* reverseList(Node* head) {
Node* prev = NULL;
Node* current = head;
Node* nextTemp;
while (current != NULL) {
nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
head = prev;
return head;
}
// 合并两个有序链表
Node* mergeSortedLists(Node* list1, Node* list2) {
Node* mergedHead = NULL;
if (!list1) return list2;
if (!list2) return list1;
if (list1->data <= list2->data) {
mergedHead = list1;
list1 = list1->next;
} else {
mergedHead = list2;
list2 = list2->next;
}
Node* tail = mergedHead;
while (list1 && list2) {
if (list1->data <= list2->data) {
tail->next = list1;
list1 = list1->next;
} else {
tail->next = list2;
list2 = list2->next;
}
tail = tail->next;
}
if (list1) tail->next = list1;
if (list2) tail->next = list2;
return mergedHead;
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
// 主函数示例
int main() {
Node* head = NULL;
// 初始化和操作链表...
return 0;
}
```
相关推荐
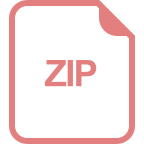
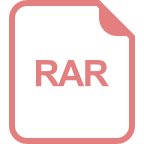
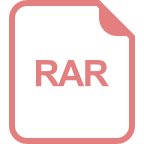














