使用Bresenham直线算法绘制颜色渐变三角形 c++代码
时间: 2024-09-30 08:03:18 浏览: 12
Bresenham算法通常用于精确地离散化直线绘制,但它本身并不直接支持颜色渐变或创建图形,因为那是更高级别的图形处理任务。不过,你可以结合Bresenham算法和简单的颜色计算来实现这个目标。
首先,你需要一个基本的Bresenham直线绘制函数,这里我们简化为一个点到点的线段绘制:
```cpp
void drawLine(int x0, int y0, int x1, int y1, std::vector<int>& colors) {
int dx = abs(x1 - x0), sx = x0 < x1 ? 1 : -1;
int dy = -abs(y1 - y0), sy = y0 < y1 ? 1 : -1;
int err = dx + dy;
while (true) {
// Bresenham's algorithm here, drawing a pixel with the current color
drawPixel(x0, y0, colors[calculateColorStep(x0, y0)]); // Assuming `drawPixel` function
if (x0 == x1 && y0 == y1) break;
if (err <= 0) {
y0 += sy;
err += dy;
}
else {
x0 += sx;
err -= dx;
}
}
}
int calculateColorStep(int x, int y) {
// Simple linear interpolation for color gradient based on x and y coordinates
// You may need to adjust this based on your specific color range and mapping
// For example, you can map x and y to a color palette index or use mathematical functions.
return (y * colorRange.width) + (x * (colorRange.height / colorRange.width));
}
```
这个例子中,我们假设`colors`是一个二维数组,表示颜色的渐变;`colorRange`是一个结构体,包含颜色范围的宽度和高度,用于生成从起始颜色到终止颜色的渐变。
请注意,这只是一个基础示例,实际应用中可能需要对颜色计算部分做更复杂的处理,并考虑如何处理边界条件以及如何将结果整合到最终的图形输出中。
相关推荐
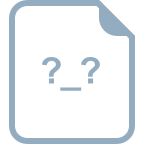
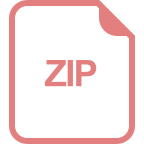
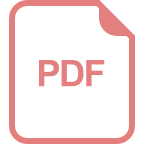














