public boolean search(String word, Node node=this.root) {
时间: 2024-10-08 17:20:33 浏览: 6
这段代码是一个Java方法,用于在一个二叉搜索树(BST)中查找特定的单词(`word`)。`Node node=this.root;`这句将当前节点初始化为根节点,假设BST有一个名为`root`的属性表示根节点。
`public boolean search(String word, Node node)`是一个公共方法,它接受两个参数:
1. `String word`: 需要查找的字符串。
2. `Node node`: 开始搜索的树节点,默认从根节点开始。
方法的功能可能是检查给定单词是否存在于BST中,通过递归遍历的方式比较每个节点的值(通常是字符串形式的整数或单词),如果找到匹配,则返回`true`;如果没有找到且遍历到叶子节点仍未找到,则返回`false`。在实际实现中,会有一个类似于这样的条件判断结构:
```java
while (node != null) {
int cmp = word.compareTo(node.value); // 比较单词和当前节点值
if (cmp == 0) return true; // 如果相等,找到了
else if (cmp < 0) node = node.left; // 如果小于当前值,向左子树搜索
else node = node.right; // 否则向右子树搜索
}
return false; // 没有找到,返回false
```
相关问题
class StreamChecker { TrieNode root; TrieNode temp; public StreamChecker(String[] words) { root = new TrieNode(); for (String word : words) { TrieNode cur = root; for (int i = 0; i < word.length(); i++) { int index = word.charAt(i) - 'a'; if (cur.getChild(index) == null) { cur.setChild(index, new TrieNode()); } cur = cur.getChild(index); } cur.setIsEnd(true); } root.setFail(root); Queue<TrieNode> q = new LinkedList<>(); for (int i = 0; i < 26; i++) { if(root.getChild(i) != null) { root.getChild(i).setFail(root); q.add(root.getChild(i)); } else { root.setChild(i, root); } } while (!q.isEmpty()) { TrieNode node = q.poll(); node.setIsEnd(node.getIsEnd() || node.getFail().getIsEnd()); for (int i = 0; i < 26; i++) { if(node.getChild(i) != null) { node.getChild(i).setFail(node.getFail().getChild(i)); q.offer(node.getChild(i)); } else { node.setChild(i, node.getFail().getChild(i)); } } } temp = root; } public boolean query(char letter) { temp = temp.getChild(letter - 'a'); return temp.getIsEnd(); } } class TrieNode { TrieNode[] children; boolean isEnd; TrieNode fail; public TrieNode() { children = new TrieNode[26]; } public TrieNode getChild(int index) { return children[index]; } public void setChild(int index, TrieNode node) { children[index] = node; } public boolean getIsEnd() { return isEnd; } public void setIsEnd(boolean b) { isEnd = b; } public TrieNode getFail() { return fail; } public void setFail(TrieNode node) { fail = node; } }
这是一个实现 Trie 树的代码,并且包含了 AC 自动机的实现,用于查询一个字符流中是否出现了 Trie 树中的某个单词。该代码中包含了主要的数据结构 TrieNode 和 StreamChecker 类,其中 StreamChecker 类包含了 query() 方法用于查询字符流,该方法的返回值为出现单词的情况。如果字符流中出现了某个单词,该方法返回 true,否则返回 false。TrieNode 类则包含了实现 Trie 树的相关方法,例如 getChild() 和 setChild() 方法用于获取和设置子节点。
@Override public boolean contains(Object o) { T element = (T) o; Node<T> x = root; int cmp; while (x != null && (cmp = element.compareTo(x.key)) != 0) { if (cmp < 0) { x = x.left; } else { x = x.right; } } return x != null; }编写测试类
好的,以下是一个简单的测试类,可以测试上述二叉搜索树的 `contains` 方法是否正确。
```java
public class BSTTest {
public static void main(String[] args) {
BST<Integer> bst = new BST<>();
bst.insert(5);
bst.insert(3);
bst.insert(7);
bst.insert(2);
bst.insert(4);
bst.insert(6);
bst.insert(8);
System.out.println(bst.contains(3)); // true
System.out.println(bst.contains(9)); // false
}
}
```
其中 `BST` 是二叉搜索树的实现类,我们可以在测试类中构建一个 `BST` 对象并插入一些元素,然后测试 `contains` 方法的正确性。在上述测试中,我们插入了 7 个元素,然后分别测试了一个存在于树中的元素 3 和一个不存在于树中的元素 9,输出应该分别为 true 和 false。
相关推荐
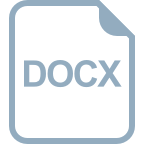
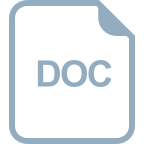
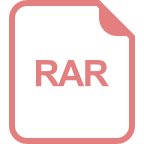












