C语言程序编写推箱子小游戏
时间: 2024-06-15 22:08:36 浏览: 197
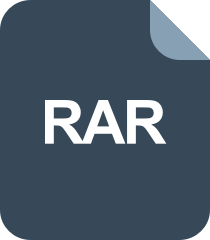
C语言编写的推箱子游戏
推箱子是一种经典的益智游戏,下面是一个简单的C语言程序编写推箱子小游戏的示例:
```c
#include <stdio.h>
#include <conio.h>
#define ROWS 5
#define COLS 5
// 定义地图
char map[ROWS][COLS] = {
{'#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', '#'},
{'#', 'O', ' ', 'X', '#'},
{'#', ' ', ' ', ' ', '#'},
{'#', '#', '#', '#', '#'}
};
// 定义玩家位置
int playerRow = 2;
int playerCol = 1;
// 游戏是否结束的标志
int isGameOver = 0;
// 打印地图
void printMap() {
system("cls"); // 清屏
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
printf("%c ", map[i][j]);
}
printf("\n");
}
}
// 处理玩家移动
void handlePlayerMove(char direction) {
int newRow = playerRow;
int newCol = playerCol;
switch (direction) {
case 'w': // 上
newRow--;
break;
case 's': // 下
newRow++;
break;
case 'a': // 左
newCol--;
break;
case 'd': // 右
newCol++;
break;
default:
return;
}
// 判断新位置是否合法
if (map[newRow][newCol] == ' ') {
// 空地,直接移动
map[playerRow][playerCol] = ' ';
map[newRow][newCol] = 'O';
playerRow = newRow;
playerCol = newCol;
} else if (map[newRow][newCol] == 'X') {
// 箱子,判断箱子后面的位置是否合法
int boxRow = newRow + (newRow - playerRow);
int boxCol = newCol + (newCol - playerCol);
if (map[boxRow][boxCol] == ' ') {
// 箱子后面是空地,移动箱子和玩家
map[playerRow][playerCol] = ' ';
map[newRow][newCol] = 'O';
map[boxRow][boxCol] = 'X';
playerRow = newRow;
playerCol = newCol;
}
}
// 判断游戏是否结束
int isWin = 1;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (map[i][j] == 'X') {
isWin = 0;
break;
}
}
}
if (isWin) {
isGameOver = 1;
}
}
int main() {
char input;
while (!isGameOver) {
printMap();
input = getch();
handlePlayerMove(input);
}
printf("Congratulations! You win the game!\n");
return 0;
}
```
这个程序使用二维数组来表示地图,玩家通过键盘输入w、s、a、d来控制上下左右移动,玩家需要将所有的箱子推到指定位置(用空地表示)。当所有箱子都被推到指定位置时,游戏结束。
阅读全文
相关推荐
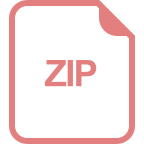
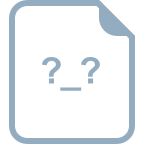
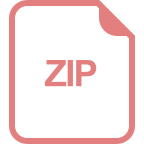
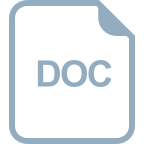
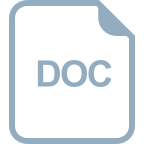
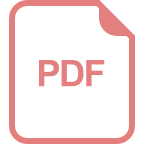
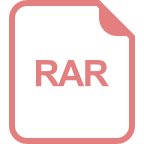
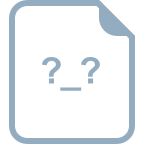
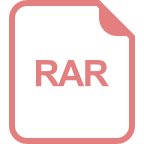
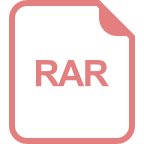
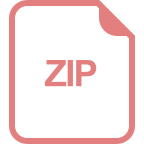
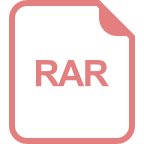
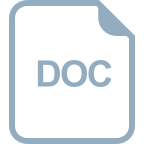
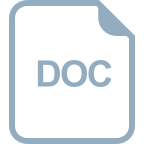
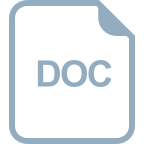