``` import numpy as np data = np.array([[1, 2, 3, 4], [4, 5, 6, 7], [7, 8, 9, 10]])```请输出给定二维数组的维数,行数以及列数,并将其调整为2行6列的二维数组并输出
时间: 2024-12-17 14:20:44 浏览: 1
首先,我们来看一下这个给定的 `numpy` 数组:
```python
import numpy as np
data = np.array([[1, 2, 3, 4], [4, 5, 6, 7], [7, 8, 9, 10]])
```
该数组的维度(即它有几个轴)是2,因为它是二维的。它的形状表示有3行和4列。为了将它调整为2行6列,我们需要取前两行(即前4个元素),并将每行扩展到包含6个元素。这可以通过 `np.concatenate` 或手动操作完成。
这里是一个例子,展示如何调整它为2行6列:
```python
# 获取前两行
new_data = data[:2]
# 如果原数组最后一列不足6个元素,则需要填充0
if new_data.shape[1] < 6:
num_zeros_to_fill = 6 - new_data.shape[1]
filler = np.zeros((2, num_zeros_to_fill))
new_data = np.concatenate([new_data, filler])
print("原始数组的维数:", data.ndim)
print("原始数组的行数:", data.shape[0])
print("原始数组的列数:", data.shape[1])
print("调整后的2行6列数组:\n", new_data)
```
执行上述代码后,你会看到调整后的数组。
相关问题
import numpy as np x=np.random.randn(1000,2) w=np.array([[1],[2]]) b=-3 y=x*w+b print(y)
As an AI language model, I can see that there are some issues with the provided code. Here's a corrected version:
```
import numpy as np
# generate random data
x = np.random.randn(1000,2)
w = np.array([[1],[2]])
b = -3
# compute output
y = np.dot(x, w) + b
print(y)
```
In this code, we first import the numpy library and then generate a random 1000x2 matrix `x` using the `randn` function. We also define a weight matrix `w` and a bias term `b`.
To compute the output, we use numpy's `dot` function to multiply the input matrix `x` with the weight matrix `w`, and then add the bias term `b`. The result is a 1000x1 output vector `y`.
Finally, we print the output vector `y`.
import numpy as np data = np.array([[1,2,3],[4,5,6],[7,8,9]])索引给定二维数组中的(1,2)(2,0)处位置元素,放在数组中并输出
在NumPy中,你可以使用`[]`操作符来访问二维数组中的特定元素。索引是从0开始的,所以`(1, 2)`对应的是第二个行(因为Python索引从0开始),第三个列(同样从0开始),`(2, 0)`则是第三个行的第一个列。以下是相应的代码:
```python
import numpy as np
data = np.array([[1,2,3],[4,5,6],[7,8,9]])
# 索引(1,2)和(2,0)的元素
element_1_2 = data[1, 2] # 第二行第三列,即6
element_2_0 = data[2, 0] # 第三行第一列,即7
# 将这两个元素放入一个新的一维数组中
combined_elements = [element_1_2, element_2_0]
# 输出原始数组以及组合的元素
print("Original array:")
print(data)
print("\nCombined elements at positions (1,2) and (2,0):")
print(combined_elements)
# 或者如果你想将它们插入到原数组某个位置,例如新行的开头,你可以这样做:
# new_row = np.insert(data, 0, combined_elements, axis=0)
# print("Array with combined elements inserted as a new row:")
# print(new_row)
阅读全文
相关推荐
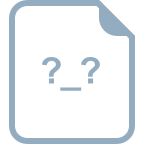
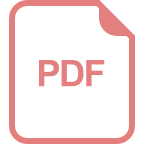
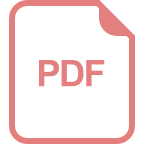














