进程调度rr调度算法 C++实现
时间: 2024-12-08 12:17:35 浏览: 21
RR(Round Robin)调度算法是一种简单的时间片轮转调度算法。在进程调度中,每个运行的进程分配一段固定长度的时间片,按照一定的顺序依次执行,当时间片结束或进程完成时,就切换到下一个进程。这种算法保证了所有进程平等获取CPU时间。
在C++中实现RR调度,你可以创建一个简单的线程池或者进程池,并维护一个队列来存储待执行的任务。下面是简化的伪代码示例:
```cpp
#include <queue>
#include <thread>
class Process {
public:
virtual void run() = 0; // 虚拟函数,子类需要实现
};
class RoundRobinScheduler {
private:
std::queue<Process*> processes;
int current_process_index;
int time_slice;
public:
void schedule(Process* process) {
processes.push(process);
}
void startNextProcess() {
if (!processes.empty()) {
current_process_index = (current_process_index + 1) % processes.size();
Process* current = processes[current_process_index];
std::thread(thread_function, current).detach(); // 创建并异步执行
// 更新时间片计时,到达时间片结束则触发下一轮调度
// ...
}
}
private:
void thread_function(Process* process) {
process->run();
// 时间片结束,将进程放回队列等待下次调度
// ...
}
};
int main() {
Process* p1 = new Process1(); // 子类Process1实现run()
Process* p2 = new Process2(); // 子类Process2实现run()
RoundRobinScheduler scheduler;
scheduler.schedule(p1);
scheduler.schedule(p2);
while (!scheduler.processes.empty()) {
scheduler.startNextProcess();
}
return 0;
}
```
在这个例子中,`Process`是一个抽象基类,`Process1`和`Process2`是它的子类,它们实现了`run()`方法。`RoundRobinScheduler`负责调度任务和管理时间片。
阅读全文
相关推荐
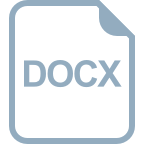
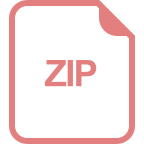
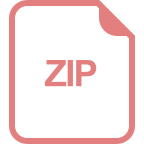
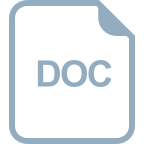
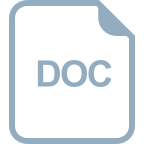


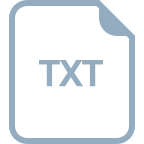
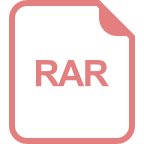
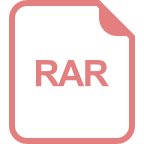
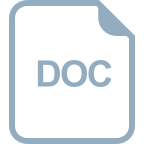






