用python写出屏蔽敏感词的代码
时间: 2024-09-11 15:10:56 浏览: 70
在Python中屏蔽敏感词可以通过简单地替换文本中的敏感词实现。这里提供一个基本的示例,使用列表存储敏感词,并使用字符串的replace方法进行替换。注意,这个简单的示例并不会处理大小写敏感和包含敏感词的情况,实际使用时可能需要更复杂的处理,比如使用正则表达式。
```python
# 定义敏感词列表
sensitive_words = ['敏感词1', '敏感词2', '敏感词3']
# 定义一个函数来屏蔽敏感词
def censor敏感词(text):
for word in sensitive_words:
# 替换文本中所有敏感词为"*",并保留原有大小写
text = text.replace(word, '*' * len(word))
return text
# 测试代码
if __name__ == "__main__":
original_text = "这是一段包含敏感词1和敏感词2的文本。"
censored_text = censor敏感词(original_text)
print(censored_text)
```
在上述代码中,`censor敏感词`函数接收一个文本字符串作为输入,遍历敏感词列表,将每个敏感词替换为相同数量的星号(*),然后返回处理后的文本。
相关问题
用python写出屏蔽敏感词的代码并运行
在Python中屏蔽敏感词可以通过多种方式实现,其中一种简单的方式是使用列表存储敏感词,并通过遍历文本内容来替换这些敏感词。下面是一个简单的Python代码示例,用于屏蔽文本中的敏感词:
```python
def filter_sensitive_words(text, sensitive_words):
for word in sensitive_words:
text = text.replace(word, '*' * len(word))
return text
# 定义敏感词列表
sensitive_words = ['敏感词1', '敏感词2', '敏感词3']
# 待处理的文本内容
text_to_filter = "这里有一些文本,其中包括敏感词1和敏感词3。"
# 调用函数屏蔽敏感词
filtered_text = filter_sensitive_words(text_to_filter, sensitive_words)
print(filtered_text)
```
在这个例子中,`filter_sensitive_words` 函数接受两个参数:`text` 是需要处理的文本内容,`sensitive_words` 是包含所有敏感词的列表。函数体内使用一个for循环遍历敏感词列表,使用`replace`方法将文本中的敏感词替换为相同长度的星号(*)。
请注意,这个代码示例在提供时并没有实际运行。在实际使用中,你需要根据实际情况定义敏感词列表,并且将上述代码放入Python环境中执行。
用python写一个敏感词替换程序
下面是一个简单的Python程序,用于将给定的敏感词替换为指定的字符串:
```python
sensitive_words = ["敏感词1", "敏感词2", "敏感词3"] # 敏感词列表
replacement = "***" # 替换字符串
def replace_sensitive_words(text):
for word in sensitive_words:
if word in text:
text = text.replace(word, replacement)
return text
if __name__ == "__main__":
text = input("请输入要替换的文本:")
replaced_text = replace_sensitive_words(text)
print("替换后的文本:", replaced_text)
```
在这个例子中,我们首先定义了一个敏感词列表`sensitive_words`和一个替换字符串`replacement`。然后定义了一个函数`replace_sensitive_words`,它接受一个文本字符串作为参数,然后遍历敏感词列表,如果在文本中找到了敏感词,则将其替换为指定的字符串。
在主程序中,我们使用`input`函数获取用户输入的文本,然后调用`replace_sensitive_words`函数进行替换,最后输出替换后的文本。
阅读全文
相关推荐
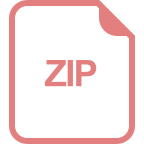
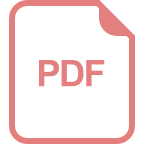
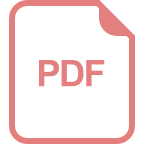









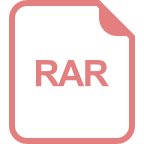
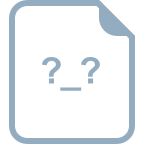
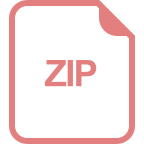

